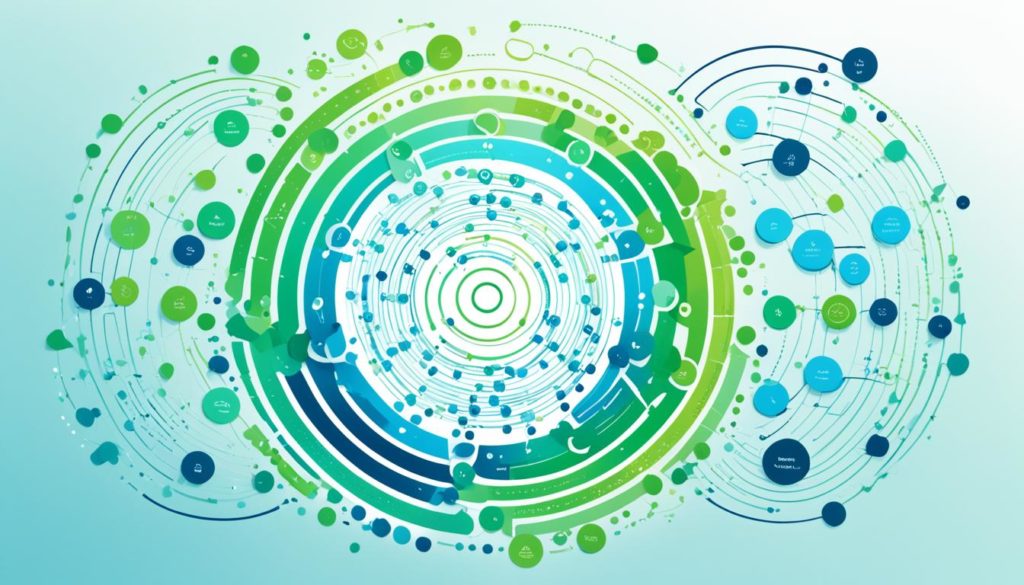
In web development, knowing React’s lifecycle is crucial. Imagine how each React component experiences three key phases—Mounting, Updating, and Unmounting. These phases are essential. They are ruled by React Lifecycle Methods, a set of tools that enhance your app. Understanding these phases leads to powerful and efficient apps that many developers worldwide trust.
The Mounting Phase is where everything starts, like a newborn’s first moment. The constructor() sets up the state of your component. The render() draws the first view of your component on the screen. Then, componentDidMount() ties up any loose ends like adding event listeners and making API calls as the component enters the DOM.
In the Updating Phase, we face change. shouldComponentUpdate() helps us decide if a re-render is needed. It’s all about making smart choices to stay agile and efficient. It’s like choosing the right dance move when the rhythm changes.
The Unmounting Phase is the end of the journey. With componentWillUnmount(), we do the final cleanup. This prevents memory leaks and ensures a smooth exit, as elegant as the entrance was.
Learning from the React Lifecycle Methods guide sharpens your skills. Understanding the lifecycle of a React component can take your apps from good to great.
Key Takeaways
- Appreciate the React lifecycle diagram as the blueprint for component behavior management throughout their lifecycle.
- Discover how constructor() and render() in the Mounting Phase lay the foundation for the component’s journey.
- Employ componentDidMount() to fasten all requisite gears and gadgets upon your component’s entrance.
- Leverage shouldComponentUpdate() in the Updating Phase to refine performance and re-render decisions.
- Navigate the intricate dance of React Lifecycle Methods to produce seamlessly functioning applications.
Understanding React Component Lifecycle
The journey through the React component lifecycle is crucial for every developer. It helps make applications robust and quick to respond. By knowing component lifecycle methods, developers gain control over a component’s behavior from start to end.
Breaking Down the Lifecycle Phases
React components go through important phases for efficiency and lifecycle management. The first phase, mounting, sets the stage. constructor() and componentDidMount() bring our components to life and to the DOM. Then, the updating phase allows components to adjust to changes, like state or props changes, with shouldComponentUpdate() and componentDidUpdate().
Last, the unmounting phase marks the end of the component’s journey in the DOM, managed by componentWillUnmount().
The Importance Of Managing State And Side Effects
Managing state and side effects is key. Knowing React component methods throughout these phases is essential. These methods help the component react to updates, from new data or user interactions. Using the right methods helps avoid memory leaks, improves user experiences, and keeps performance high.
React Lifecycle Methods Overview
The lifecycle starts strongly with the mounting phase, preparing components for the user. The updating phase, triggered by props or state updates, is crucial. It adjusts components’ outputs to ensure the UI matches the component states in real-time.
To apply these ideas, looking at a detailed guide on React component lifecycle methods helps. Understanding these methods boosts performance and exploits React’s potential for superior applications.
Exploring the Mounting Phase
The mounting phase in React marks the beginning of a component’s journey. This initial stage is crucial as it lays the foundation for the component’s behavior and updates. By using key React component methods, we ensure a strong start for the component.
The constructor starts this phase, setting the stage for state setting and method binding. static getDerivedStateFromProps() follows, allowing state adjustments based on initial props. This ensures the component reacts to changes even before it’s seen. Then, render() creates a virtual DOM snapshot, previewing the upcoming screen content.
After the component is rendered, componentDidMount() comes into play. This method is essential for operations needing DOM interaction or data fetching. It helps smoothly integrate with other JavaScript frameworks and libraries.
This mounting phase is similar for both class-based and Hooks-based components, but their execution differs. Class-based components depend on componentDidMount for after-mount side-effects. On the other hand, Hooks-based components use the useEffect Hook for similar outcomes, but in a more straightforward manner.
Knowing about React component methods during the mounting phase boosts our ability to build effective React apps. It also enhances performance right from the start. Taking care to correctly initialize components sets a strong basis for handling updates and re-renders.
To learn more about this, check out a comprehensive guide on React component lifecycle. It offers deeper insights into managing component life from start to end.
By understanding React component mount and methods, we improve our coding. It helps our apps manage complex states and interactions better. So, let’s keep building and learning, ensuring our React components start off right.
The Significance of the Updating Phase in React
Exploring the React component’s lifecycle, it’s clear the updating phase is key. It boosts app performance and user interaction. Here, React Component Methods and Lifecycle Hooks are critical. They help decide if and how components should update.
Let’s look at how methods like shouldComponentUpdate(), getSnapshotBeforeUpdate(), and componentDidUpdate() impact this phase. They are game-changers.
Making the Most of shouldComponentUpdate()
The shouldComponentUpdate() method is a checkpoint for updates. It compares current props and state with new ones. If no update is needed, it stops the rendering. This saves resources and improves performance.
The Role of getSnapshotBeforeUpdate() and componentDidUpdate()
getSnapshotBeforeUpdate() takes a snapshot before the DOM updates. It’s great for keeping things like scroll position. Then, componentDidUpdate() comes into play. It allows DOM interactions after updates, like fetching data or using APIs. It keeps the component responsive to users.
Method | Purpose | Phase |
---|---|---|
shouldComponentUpdate() | Decides if re-rendering is necessary | Pre-Update |
getSnapshotBeforeUpdate() | Captures DOM state | Just Before Update |
componentDidUpdate() | Operates on DOM post-update, fetches data | Post-Update |
Using these React Lifecycle Hooks makes the update phase smooth. This makes apps more efficient and responsive. Focusing on these methods will help your React apps stay strong and quick.
React Lifecycle Methods: Component Unmounting
In our journey through React Lifecycle Methods, we focus on the last phase: unmounting. This is when a component says goodbye. The componentWillUnmount() method is like a stage manager. It makes sure everything is cleared away. This step is key for keeping React apps running smoothly. It stops memory leaks that slow down the app.
The work done during the componentWillUnmount() phase is crucial. We stop timers, cancel network requests, and remove event listeners set up in componentDidMount(). This keeps the app’s memory in check and saves resources. It’s vital for the app’s long-term health. For more info, check out this deep dive into React component unmounting.
It’s important for developers to use React Lifecycle Methods wisely, especially unmounting. We make sure components vanish cleanly when not needed. By doing this, we keep our applications efficient and reliable. The unmounting phase might not be flashy, but it’s crucial. It ensures that every component’s exit is as smooth as its entry.
FAQ
What Are React Lifecycle Methods?
React Lifecycle Methods are steps that happen at key times in a component’s life in a React app. Developers can use these times to do specific tasks. These tasks happen when a component starts, updates, and stops being used.
Can You Explain the React Component Lifecycle Phases?
Sure! The React component lifecycle has three phases: mounting, updating, and unmounting. Mounting is when the component is made and placed in the DOM. Updating happens when changes in the component’s state or props require it to render again.
Unmounting is when the component is taken out of the DOM. This is when you need to clean up to avoid memory leaks and other problems.
What Happens During the Mounting Phase?
In the mounting phase, a React component is created. It starts with the constructor() to set up state, then getDerivedStateFromProps() updates state based on props. Next, render() makes the virtual DOM.
Finally, componentDidMount() does tasks needing the DOM or external data, like API calls.
How Crucial Is Managing State and Side Effects in React?
It’s very important to manage state and side effects in React apps. State controls the component’s data and behavior. Side effects can impact other parts of your app.
Good management keeps the app smooth, fast, and easy to handle.
What Is shouldComponentUpdate() and Why Is It Important?
shouldComponentUpdate() helps during updates. Developers use it to decide if a component should render again. It’s key for keeping an app fast by avoiding needless re-renders.
What Are getSnapshotBeforeUpdate() and componentDidUpdate() Used For?
getSnapshotBeforeUpdate() is used right before an update. It can grab data from the DOM to use later. Then, componentDidUpdate() runs right after an update. Developers can work with the updated DOM or get more data if needed.
When Is componentWillUnmount() Called and Why Is It Important?
componentWillUnmount() happens before a component is gone from the DOM. It’s time to clean up resources like timers or event listeners. This avoids slowing down the app or using too much memory.
What Is the React Lifecycle Diagram?
The React Lifecycle Diagram shows the order and timing of lifecycle methods. It shows how components are made, updated, and removed. This helps developers know when and why to use different methods.
How Do React Lifecycle Hooks Relate to Lifecycle Methods?
React Lifecycle Hooks let you use React’s state and lifecycle features in function components. They offer an alternative to class components’ lifecycle methods. The useEffect hook, for instance, can handle side effects and take over for componentDidMount, componentDidUpdate, and componentWillUnmount.
Future App Studios is an award-winning software development & outsourcing company. Our team of experts is ready to craft the solution your company needs.