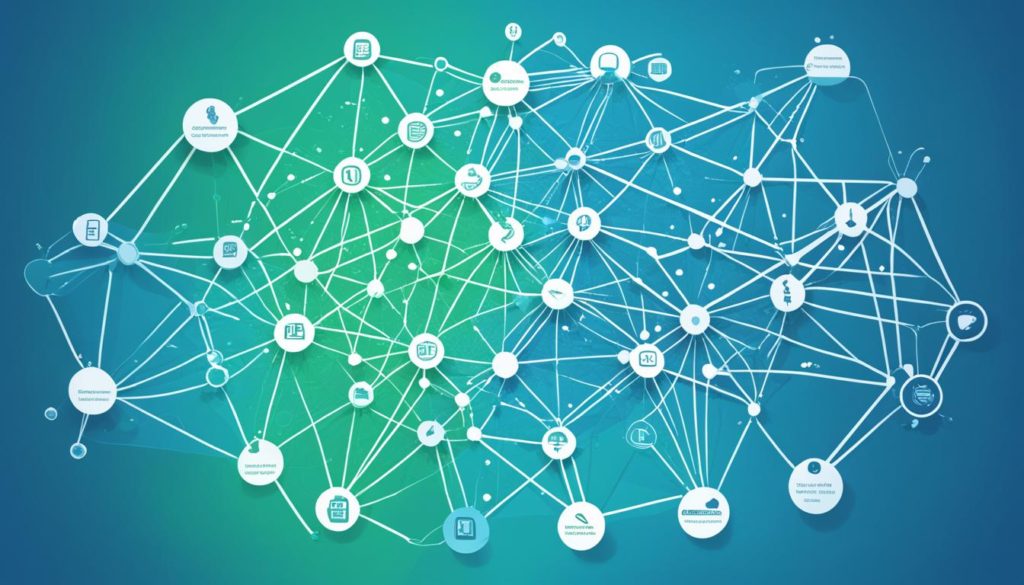
Did you know that over half of the slowdowns in apps come from blocking calls in synchronous programming? This major fact shows the big difference between synchronous vs asynchronous programming in making software. Knowing when to use each type can make our apps work better and respond faster.
Synchronous programming means running code step by step. It’s simple and easy to understand. But, it can slow things down when you have tasks that wait on each other. Asynchronous programming does many things at once. This can make our apps work faster and more smoothly, particularly when they’re doing a lot of waiting, like on the web.
The synchronous way is easy and reliable, while asynchronous programming lets us do more things at the same time. This can make apps better for users. The choice between synchronous and asynchronous depends on what the app needs. This includes how it flows, waits, and how complex it is.
Key Takeaways
- Synchronous programming executes code sequentially.
- Asynchronous programming allows for concurrent execution.
- Over 50% of app performance issues stem from blocking calls in synchronous code.
- Understanding both paradigms is essential for optimizing application performance.
- Choose the appropriate model based on factors like latency, complexity, and scalability.
Understanding Synchronous Programming
Synchronous programming is a traditional way of coding where tasks happen one after the other. This method makes it simpler to understand how the code works. It’s great for easy or small projects.
Key Characteristics of Synchronous Programming
Synchronous programming mainly relies on tasks happening in sequence. One task finishes before the next starts, creating an orderly process. This common approach, used in languages like C, Java, and Python, suits certain apps well. It works best where tasks need to happen in a specific order.
Real-Life Examples of Synchronous Programming
A basic calculator is a good example of synchronous programming. It does math operations one by one. This ensures each step is finished properly before moving on. Reading a file before using its data in scripts is another example.
Advantages of Synchronous Programming
Synchronous programming has its pluses. First, its step-by-step nature makes code easier to handle. Developers find this style user-friendly for smaller tasks. Also, it guarantees tasks are done in order, which helps in simple projects.
Disadvantages of Synchronous Programming
However, synchronous programming isn’t perfect. It struggles with long tasks, like fetching database data or web requests. This waiting can slow everything down, making for a bad user experience. So, it’s less ideal for projects needing fast, concurrent actions.
For more info, check out this Stack Overflow discussion on synchronous versus asynchronous execution. It’s key to know which to use for your project.
Understanding Asynchronous Programming
Asynchronous programming is key in modern software development. It lets tasks run at the same time, boosting app performance. We use non-blocking operations and event-driven programming for better resource use and user experience.
Key Characteristics of Asynchronous Programming
It’s known for non-blocking operations. Tasks can run on their own, without waiting on each other. This way, many operations can start at once without stopping each other.
An example of asynchronous programming is getting data from APIs in web development. This keeps the app working smoothly while waiting for data, making the user experience better.
Real-Life Examples of Asynchronous Programming
In web applications, managing file uploads uses asynchronous techniques. Users can keep using the site while their files upload in the background.
Another example is in JavaScript, using functions like fetch to request server data. This doesn’t stop the main thread.
Advantages of Asynchronous Programming
Its main plus is improving performance. Tasks run at the same time, avoiding delays. This makes for a responsive interface when handling big tasks.
Asynchronous frameworks also boost scalability. They let apps do more at once without overloading. Using frameworks like Node.js helps create fast network applications.
Disadvantages of Asynchronous Programming
However, asynchronous programming can make code harder to manage. Debugging is tough due to the tasks not going in order. It’s crucial to manage callbacks and async syntax right to avoid problems.
Even though it makes apps efficient and responsive, it needs a good understanding of asynchronous patterns and handling concurrency well to dodge issues.
Synchronous vs Asynchronous Programming
When we talk about synchronous vs asynchronous programming, it’s important to note their differences. Each style has its own benefits. They affect how fast an app runs and how easily developers can work.
Key Differences
Synchronous and asynchronous programming differ mainly in how they run tasks and if they wait for tasks to finish.
- Synchronous Programming: It follows a step-by-step path. One task finishes before another starts.
- Asynchronous Programming: It does many tasks at the same time. This can make things work faster in lots of cases.
In synchronous programming, one task must finish before another can start. This makes it simple to follow but can slow things down. On the other hand, asynchronous programming lets different tasks go at their own pace without waiting. This can speed things up but might make finding problems harder.
When to Use Synchronous Programming
Choosing synchronous programming is good for certain situations. It works well when:
- Tasks need to happen in a specific order. For example, making videos or loading web pages in a way that’s good for SEO.
- When it’s important to find and fix errors easily.
When to Use Asynchronous Programming
Knowing when to pick asynchronous programming is key. It’s great for:
- Tasks like working with databases where you don’t have to wait for one task to end to start another.
- Creating dynamic dashboards that do many things at once. This makes them work faster and better.
Aspect | Synchronous Programming | Asynchronous Programming |
---|---|---|
Execution Model | Sequential | Concurrent |
Blocking Behavior | Blocks subsequent tasks | Non-blocking |
Debugging Ease | Easy | Complex |
Performance | Slower | Faster |
Knowing the differences and when to use each programming style helps us improve our apps. It lets us choose the best approach for speed and reliability.
Converting Synchronous Code to Asynchronous Code
Switching synchronous code to asynchronous can boost your app’s speed and response. It’s great for lengthy tasks, like I/O jobs. Let’s look at how this is done.
Identify Operations to Convert
We first find what slows us down, often tasks that wait on something else. Like reading files or making network requests. Turning these into asynchronous tasks speeds things up.
Choosing Asynchronous Methods
After pinpointing what needs changing, we pick how to do it asynchronously. Options range from callbacks and promises to async/await in Node.js. Moving from synchronous file operations to asynchronous ones can make a big difference.
Refactoring Code
We then change our code to work asynchronously. Maybe we switch to promise-based functions or use async/await. It’s about keeping our app working the same but faster.
Handling Asynchronous Results
Dealing with the outcomes of async tasks is key. We might use .then() and .catch() for promises, or try/catch with async/await. It helps our app stay solid and recover smoothly from issues.
Following these steps changes our code for the better. Making it efficient and quick improves our app’s performance overall.
Common Use Cases of Synchronous and Asynchronous Programming
Grasping the differences between synchronous and asynchronous communication is key for spotting when to use each. Synchronous code runs in order, waiting for the step before to end. This suits tasks needing a set sequence or intense computing. Examples are real-time apps, game making, processing data, and rendering web pages or videos.
Asynchronous programming lets many processes happen at once without waiting on each other. It shines in high concurrency and fast response needs. Use it for big databases and updating complex dashrooms instantly. It’s used in JavaScript, Python’s asyncio, RxJS, and Node.js for effective multitasking, better resource use, and snappier interfaces.
We’ve outlined some classic uses for each method. Synchronous fits for tasks requiring precision and exact order, like in embedded systems or event programming. Asynchronous is ideal for background jobs or separate tasks. Examples include data loading, long computations, and keeping UIs swift.
The choice between synchronous and asynchronous talks a lot about trade-offs. While synchronous code is simpler to write and fix, it might lag in complex tasks. On the flip side, asynchronous code is quick and scales well but can be tricky with errors and callback management. noticed
Choosing the right method depends on your project’s specific needs. Knowing the strengths and challenges of each can boost both efficiency and user happiness.
Conclusion
Choosing between synchronous and asynchronous programming depends on your project’s needs. Synchronous programming is great for simple tasks. It’s predictable and easy to grasp. This makes it good for beginners or when you need things to happen in order. Asynchronous programming is better for complex projects. It speeds up processing, improves user experiences, and manages resources better. But, it needs careful coding to avoid pitfalls.
To pick the right approach, understand how each programming model works. This knowledge helps in optimizing code and building strong applications. By applying programming best practices, we choose the right paradigm for each task. This leads to better code and improved performance. We must weigh the pros and cons of each model carefully. This helps us make choices that fit our project’s goals and requirements.
The choice between synchronous and asynchronous programming depends on different factors. Both options have their benefits and limitations. Knowing how to use both effectively is key to building efficient and scalable apps. It helps us meet our programming and project targets more successfully.
FAQ
What is the difference between synchronous and asynchronous programming?
Synchronous programming runs code one step at a time. Each step must finish before the next one starts. Asynchronous programming can do many things at once. It does not wait for a task to finish before starting another one.
What are the benefits of asynchronous programming?
Asynchronous programming lets multiple tasks run at the same time. This makes things more efficient and keeps the program responsive. It’s great for tasks that need updates in real-time or manage a lot of data.
What are the advantages of synchronous programming?
Synchronous programming is easier to use and fix because it follows a simple order. It’s best for beginners or when tasks must be done one after another.
When should I use synchronous programming?
Use synchronous programming for simple or step-by-step tasks. For example, calculations in a calculator app or making web pages friendly for search engines.
Can you provide an example of asynchronous programming?
An example is getting data from APIs in web development. While the data is being fetched, the app can still work. This keeps the app smooth for users.
Another example is uploading files without stopping user interaction.
What are the disadvantages of asynchronous programming?
Asynchronous programming can be tricky and hard to debug. Keeping track of multiple tasks and managing errors is more complex than with synchronous programming.
What steps are involved in converting synchronous code to asynchronous code?
First, find the parts of code that slow down execution. Then, choose the right asynchronous methods like callbacks or promises. Next, change the code to use these methods. Finally, make sure the new code works well after changes. In Node.js, this might mean using asynchronous file reading instead of synchronous.
How do synchronous and asynchronous programming impact scalability?
Asynchronous programming improves scalability by letting many tasks run at the same time. This is good for apps with lots of data work. Synchronous programming is simpler but can slow down growth if the app gets too busy.
Future App Studios is an award-winning software development & outsourcing company. Our team of experts is ready to craft the solution your company needs.