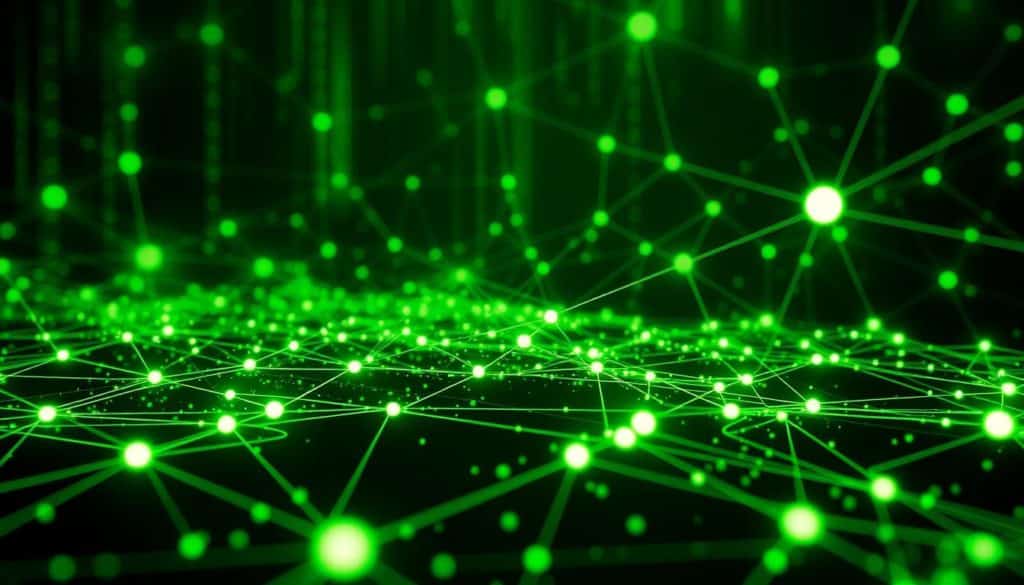
Did you know the “ws” library for Node.js is a top pick for WebSocket apps? It has over 20,000 stars on GitHub. This shows how trusted and reliable it is for making real-time apps. Let’s dive into how Node.js WebSocket can change your server-side JavaScript projects with its real-time chat features.
Key Takeaways
- Understanding WebSocket and its role in real-time communication.
- Efficient use of Node.js WebSocket for superior performance and scalability.
- The strength of the Node.js WebSocket community and ecosystem.
- Step-by-step guidance on setting up a basic Node.js WebSocket server.
- Insights into integrating WebSocket in real-time applications such as chat and live data streaming.
Understanding WebSockets and Real-Time Communication
The WebSocket protocol is a key tool for real-time communication. It lets data flow both ways, making updates and notifications instant. This is thanks to its ability to keep a connection open between a browser and a server.
The WebSocket API is at the heart of this tech. It helps set up and manage WebSocket connections. Tools like the MessageEvent and CloseEvent are crucial for sending messages and ending connections.
Understanding HTTP headers is vital when using the WebSocket protocol. By using the WebSocket API, users get a more dynamic and responsive experience. This is thanks to the smooth interactive communication session.
Why Choose Node.js WebSocket for Your Project?
Node.js WebSocket is a top choice for projects needing fast, efficient networking and real-time features. It uses a non-blocking I/O model that works well with JavaScript. This makes applications run smoothly and quickly.
Efficiency and Performance
Using WebSocket libraries with Node.js means a constant connection between the server and client. This connection is key for real-time apps, offering great efficiency and speed. It helps us handle tasks fast, giving users less wait time and better real-time chats.
Scalability
Node.js is great at handling many connections at once, which is perfect for growing server setups. With WebSocket, apps can grow easily as more people use them. This means better use of resources and a better experience for users.
Community and Ecosystem
Node.js WebSocket also has a strong community and a big ecosystem. There are many WebSocket libraries out there, like Socket.IO and WebSocket-Node. These libraries offer powerful tools and support for developers. The big community means there are always updates and resources available, making it easier to build and keep up real-time apps.
Setting Up a Basic Node.js WebSocket Server
Setting up a basic Node.js WebSocket server is key for adding real-time features. It’s easy and can be done with npm packages. This guide will show you how to install the WebSocket library, create a server, and manage WebSocket events.
Installing WebSocket Library
First, make sure you have Node.js server installation done on your machine. Then, install the WebSocket library with npm by typing this in your terminal:
npm install websocket
This library makes setting up and managing your WebSocket server easier. It helps with real-time data exchange.
Creating a Simple WebSocket Server
After installing the library, creating a WebSocket server is simple. Here’s a basic example to start with:
const WebSocketServer = require('websocket').server;
const http = require('http');
const server = http.createServer((request, response) => {
response.writeHead(404);
response.end();
});
server.listen(8080, () => {
console.log('Server is listening on port 8080');
});
const wsServer = new WebSocketServer({
httpServer: server
});
wsServer.on('request', (request) => {
const connection = request.accept(null, request.origin);
connection.on('message', (message) => {
console.log('Received Message:', message.utf8Data);
connection.sendUTF('Hello Client!');
});
connection.on('close', (reasonCode, description) => {
console.log('Client has disconnected.');
});
});
This code sets up an HTTP and a WebSocket server. Clients can connect and send messages. You can customize this to fit your project’s needs.
Handling WebSocket Events
Managing WebSocket events is crucial for WebSocket event management. In our example, we handle ‘request’, ‘message’, and ‘close’ events. This makes managing connections and data transfers smooth. For more complex setups, check out tutorials like the one on Postman.
Advanced Features and Configurations of Node.js WebSocket
Node.js WebSocket has many advanced server features that boost performance and reliability. It can handle fragmented messages, keeping data safe during transmission.
Customizing settings like frame size and message fragmentation lets us tailor the server to our needs. This makes our server efficient and ready for various data streams.
For security, we can set up secure TLS connections to protect our data. Keep-alive pings also help keep connections stable, avoiding timeouts and ensuring we’re always available.
Feature | Description |
---|---|
Fragmented Message Handling | Ensures data integrity by combining message fragments. |
Frame Size Customization | Allows configuration of frame size for data packets. |
Secure TLS Connections | Establishes encrypted channels for safe communication. |
Keep-Alive Pings | Maintains stable connections by preventing timeouts. |
Using these advanced features, we can build a strong WebSocket setup for real-time apps. This ensures top performance and lets us customize solutions for our needs.
Integrating WebSocket in Your Real-Time App
WebSockets have changed how we make real-time apps. They let us create chat platforms and data streaming services that update instantly. This makes communication smooth and fast.
Example: Real-Time Chat Application
WebSocket is great for chat apps. Imagine a chat app that lets users talk in real-time without delay. With WebSocket, messages go back and forth super fast. This makes chatting fun and easy.
For a detailed guide on making such an app, check out this Node.js WebSocket chat tutorial.
Example: Live Data Streaming
WebSocket is also key for live data streaming. Services use it to send updates on things like stock prices or sports scores live. WebSockets send data from server to client quickly and efficiently.
For example, it’s great for financial apps that need to update stock prices fast. This keeps users informed and gives them an edge in the market.
Here’s a look at the benefits:
Feature | Chat App Development | Live Data Streaming |
---|---|---|
Communication Speed | Instantaneous | Continuous updates |
User Engagement | High | Very high |
Data Transfer | Bidirectional | Unidirectional |
Adding WebSockets to apps makes them better and more engaging. It’s especially useful for chat apps and live data streaming. Both benefit greatly from WebSocket integration.
Conclusion
Node.js WebSocket has become a crucial tech for making apps work fast and grow big. It combines an event-driven JavaScript engine with a strong networking protocol. This gives developers what they need for making apps that interact with users in real time.
We talked about how Node.js WebSocket helps with speed, performance, and growing big. It also has a strong community backing it up. By setting up a simple WebSocket server and adding it to real apps like chat apps and live data streams, we can make apps that talk to users in real time easily.
As we keep learning and using WebSocket, it’s clear it will keep leading the way in making new networked apps. Whether you’re an expert or new to coding, using Node.js WebSocket gives you a powerful tool. It helps you make apps that are lively and keep users engaged in real time.
FAQ
What is Node.js WebSocket?
Node.js WebSocket lets a client’s browser talk to the server in real-time. It’s great for projects needing quick responses. This is because it supports two-way, event-driven communication.
Why should we use WebSocket for real-time apps?
WebSockets are perfect for real-time apps like chat, live updates, and streaming. They cut down on the need for constant checking, which means less delay and better performance.
What makes the “ws” library popular among developers?
The “ws” library is loved for being simple, fast, and well-tested. It’s open-source and free to use, thanks to the MIT license. Plus, it has a big community backing it up, shown by its many stars and forks on GitHub.
How do WebSocket events like CloseEvent and MessageEvent work?
WebSocket events like CloseEvent and MessageEvent are key to handling WebSocket connections. CloseEvent is for when a connection ends, and MessageEvent is for incoming messages. This lets the server answer right away.
What are some advanced features of Node.js WebSocket?
Node.js WebSocket has cool features like handling broken messages, setting frame sizes, secure TLS connections, and keep-alive pings. These help servers work better and stay reliable by offering more control.
How to set up a basic WebSocket server in Node.js?
To start a basic WebSocket server, first install a WebSocket library with npm. Then, create a server and define how it handles WebSocket events like messages and connections.
What are the benefits of using Node.js WebSocket for real-time apps?
Using Node.js WebSocket brings many perks. It ensures fast, efficient real-time communication thanks to Node.js’s design. It also scales well for lots of connections and has a strong library and community support.
Can we integrate WebSocket for building a real-time chat application?
Yes! WebSocket is great for chat apps. It lets users talk to the server instantly, making the experience smooth and interactive.
How does WebSocket help in real-time data streaming?
WebSocket is perfect for streaming data in real-time. It keeps data flowing constantly between the client and server. This means users get updates right away.
What is the significance of community support and ecosystem for Node.js WebSocket?
A strong community and ecosystem for Node.js WebSocket offer lots of resources and libraries. This makes building real-time apps easier and more efficient for developers.
Future App Studios is an award-winning software development & outsourcing company. Our team of experts is ready to craft the solution your company needs.