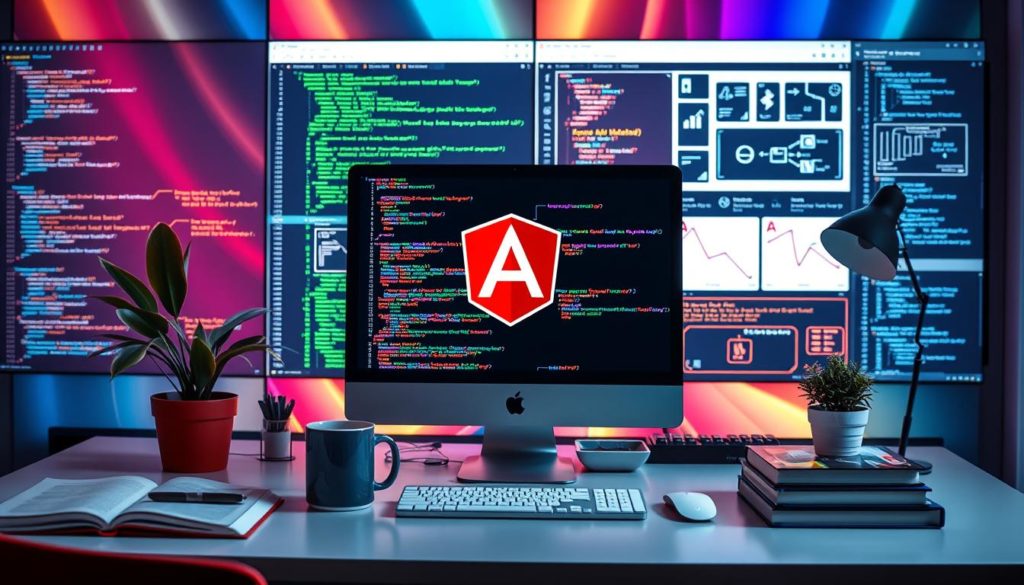
Did you know over 500,000 websites use Angular as their main framework? This tool is huge and very popular. Big names like Google Cloud Console and Microsoft Office Web Apps rely on Angular for smooth user experiences.
Learning Angular means diving into complex ideas and hands-on examples. You might use a `GreetingComponent` for dynamic greetings or a `DataService` for fetching data. Angular is a full package for building strong web apps. Let’s explore these ideas to boost our skills and make top-notch web applications.
Key Takeaways
- Angular is used by over 500,000 websites, showcasing its popularity.
- Angular development services provide the tools needed for advanced web applications.
- Key platforms like Google Cloud Console and Microsoft Office Web Apps use AngularJS services.
- Understanding Angular service providers is crucial for building robust web apps.
- Dynamic components and data services enhance the functionality of Angular applications.
Introduction to Angular Services
Angular is known for its flexibility, speed, and strong features. It’s a key part of making dynamic web apps. In this Angular service tutorial, we’ll see how Angular services help manage tasks like getting data and connecting to networks across different parts of an app.
To start with Angular services, you need to know about dependencies like Node.js and npm. Using Angular services makes your code easier to use and keep up with changes. The Angular CLI is a big help in setting up projects. It offers tools like routing and SCSS for easier development.
Components like mycomp.component show off Angular’s ability to keep things organized and scalable. Services like myserv.service highlight how Angular keeps different parts of your code separate. This makes your code easier to use again. By following this tutorial, you’ll learn how to build strong web apps with Angular services.
Creating and Injecting Angular Services
Angular services are key in making web apps strong. They help share data and manage logic across components. This part explains how to make and use Angular services to improve our app’s performance.
Using Angular CLI
To make a service in Angular, the Angular CLI is very helpful. By typing ng g s myserv
, we get a service template. This step is crucial for organizing and planning services.
After making the service, we need to add it to components to use its features. We can bring the service into a component and add it through the constructor. This is a simple way shown in many Angular service examples:
import { MyService } from './myserv.service';
@Component({
selector: 'app-example',
templateUrl: './example.component.html',
styleUrls: ['./example.component.css']
})
export class ExampleComponent {
constructor(private myService: MyService) { }
// Method using the service
someMethod() {
this.myService.serviceMethod();
}
}
Dependency Injection in Angular
Dependency Injection (DI) is a key part of Angular’s service setup. It lets us create objects that other classes need, making the code easier to test and more modular.
Angular uses Dependency Injection to manage services well across the app. We can list services in a module or a component’s providers. This keeps the code clean and organized by sharing and reusing services as needed.
Angular CLI Command | Action | Example |
---|---|---|
ng generate service | Creates a service | ng g s myserv |
@Injectable decorator | Adds metadata | @Injectable({ providedIn: ‘root’ }) |
Providers Array | Registers services | @NgModule({ providers: [MyService] }) |
Benefits of Using Angular Services
Using Angular services in our web apps has many advantages. These benefits show up as we see how Angular service providers make apps stronger and keep code clean and organized. Let’s look at the main benefits of using Angular services.
Reusability
Angular services are great for reusing code. By putting business logic in services, we can use the same code in different parts of an app. This makes development faster and keeps code clean. Angular service providers help us manage reusable code easily.
Separation of Concerns
Angular development services help separate different parts of an app. Each part does its own job, making the app easier to understand and maintain. Services handle business logic, while components deal with user interactions and views. This makes apps more organized and scalable.
For more on why services are key in Angular, check out this in-depth guide.
Improved Testability
Services make testing Angular apps easier. Putting business logic in services makes it simpler to test. We can test each service on its own, away from components. This helps find and fix bugs faster, making apps more stable and reliable.
Benefits | Description |
---|---|
Reusability | Facilitates code reuse across various parts of the application. |
Separation of Concerns | Delegates responsibilities clearly, resulting in a clean architecture. |
Improved Testability | Allows isolated unit testing, enhancing bug detection and resolution. |
Best Practices for Angular Service Development
To make Angular services strong and efficient, we must follow best practices. These rules help our services grow, stay easy to keep up, and work well. Let’s look at the main Angular service best practices we should think about.
Single Responsibility Principle
The Single Responsibility Principle is key. Each service should focus on one job or task. This makes our code easy to manage and understand. For example, a data service should only deal with getting data, not other tasks.
This way, fixing problems is easier and we can use the same code in different places. It makes our app better.
Use of Observables
Using Observables from the RxJS library is important for handling tasks that take time. Observables help us manage data streams and events well. They make our Angular services more flexible and able to grow, making our app better at handling user actions and server replies.
Lazy Loading Services
Lazy loading is a great way to make our app run faster. It loads services only when they are needed, cutting down on startup time and saving resources. This is especially useful for big apps where not everything needs to start right away.
Following these Angular service best practices helps us build apps that work well, are easy to keep up, and can grow. By focusing on one task per service, using Observables, and lazy loading, our Angular services will be strong and efficient.
Advanced Techniques in Angular Service Architecture
Understanding Angular’s full potential means learning about advanced service architecture techniques. This includes using lazy loaded modules through Angular routing. This boosts app performance and improves user experience. Version 18 of Angular brings new features like performance boosts, TypeScript 5.0 support, and state management tools, making the service architecture stronger.
In this Angular service tutorial, we explore key techniques for developers. These techniques help build apps that are scalable and easy to maintain. For example, lazy loading modules makes apps more efficient by loading only what’s needed, which is crucial for Angular service architecture.
Developers can use these advanced techniques by following these strategies:
- Using tools like NgRx or Akita for efficient state management
- Improving app performance with Ahead-of-Time (AOT) compilation
- Utilizing Angular’s features with lazy loading and route guards
Let’s look at how Angular version 18 compares to earlier versions:
Feature | Angular 16 | Angular 18 |
---|---|---|
Performance Optimizations | Basic Improvements | Advanced Improvements |
TypeScript Support | TS 4.0 | TS 5.0 |
State Management | Basic State | Enhanced State |
Conclusion
In this article, we’ve looked at AngularJS services and their key role in making web apps work well. We learned how to use the Angular CLI for creating services and how to design apps with good architecture.
Mastering these ideas can take your development skills to the next level. You can make apps that are as good as Google Cloud Console. Understanding Angular services not only boosts our technical skills but also helps us grow professionally in the changing tech world.
By using dependency injection and the Singleton Pattern, we get better at making apps that are easy to use and efficient. As we keep learning about AngularJS services, let’s keep focusing on the best ways to do things. This will help us make apps that meet today’s digital needs and succeed in the tech industry.
FAQ
What are Angular services and why should we use them?
Angular services are special objects that hold reusable code. They help make our code modular and easy to maintain. They separate different parts of our code, making it reusable and easier to test.
How do we create an Angular service using Angular CLI?
To create an Angular service with Angular CLI, use the command `ng g s myserv`. This makes a service called `myserv`. We can then easily add it to our components.
What is Dependency Injection in Angular?
Dependency Injection (DI) is a pattern used by Angular to make our code more modular and testable. It helps manage service instances by injecting them into our components.
How does Angular service architecture contribute to enhanced reusability?
Angular services make our code reusable by letting it be used in different parts of the app. This makes our code consistent and cuts down on repetition. It makes our apps easier to keep up and grow.
What benefits do Angular services provide in terms of Separation of Concerns?
Angular services help separate different tasks into different service classes. This makes our code cleaner, with logic and templates kept separate.
How do Angular services improve testability?
Angular services make testing easier by letting us test code units on their own. This way, we can find and fix bugs more easily.
What is the Single Responsibility Principle in the context of Angular services?
The Single Responsibility Principle means a service should handle just one task. This makes our services simpler, easier to test, and maintain.
What role do Observables play in Angular services?
Observables, from the RxJS library, are key for handling data streams and asynchronous tasks in Angular services. They help us work with data and detect changes effectively.
How does Lazy Loading Services improve Angular application performance?
Lazy Loading Services make apps faster by loading services only when needed. This cuts down the time it takes to load and uses resources better.
What are some advanced techniques in Angular service architecture?
Advanced techniques include using Angular routing for lazy loading modules and state management solutions. These improve performance, scalability, and user experience.
Future App Studios is an award-winning software development & outsourcing company. Our team of experts is ready to craft the solution your company needs.