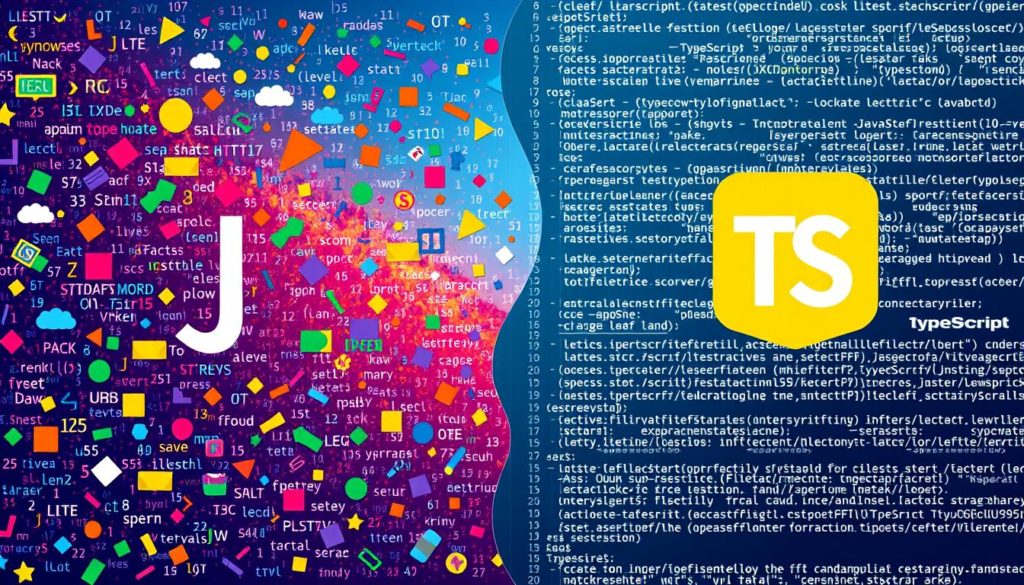
Did you know over 97% of websites use JavaScript? As web developers, we often work with JavaScript. It’s key in web design and server-side applications through Node.js. For complex projects, many turn to TypeScript. It’s a powerful JavaScript superset that helps prevent errors and makes projects easier to maintain.
JavaScript and TypeScript have unique benefits for different needs. If you’re working on small tasks, JavaScript might be the better choice. But for big projects, TypeScript’s strong type safety and error checking are a must. It’s important to know the main differences to pick the right tool for your project.
Key Takeaways
- JavaScript is used on over 97% of websites, showing its wide use in web development.
- TypeScript builds on JavaScript with static typing, perfect for big projects.
- JavaScript lets you code quickly with flexible typing, without strict type rules.
- TypeScript’s compile-time checks can spot errors early, avoiding runtime problems.
- For simple tasks, JavaScript is faster to start and easier to use.
- TypeScript takes more effort to learn but offers better error handling and upkeep.
- Choosing between JavaScript and TypeScript depends on your project’s needs and goals.
For more details on the differences, check out this article on the differences between JavaScript and TypeScript. Using each language’s strengths can greatly improve your project’s success.
Understanding JavaScript
JavaScript is a key language for making web pages interactive. It lets us add cool stuff to websites. JavaScript is flexible and works on all modern web browsers. This makes it a must-have for creating engaging online experiences.
Core Features
JavaScript has some main features:
- Interpreted Execution: It doesn’t need compiling, which speeds up development.
- Dynamic Typing: You can store any type of value in a variable.
- Event-driven Programming: It makes websites react to what users do.
- Asynchronous Operations: It handles tasks that run at the same time well.
- Broad Browser Support: It works on all web browsers.
Advantages of JavaScript
JavaScript has many benefits for developers:
- Simplicity: It’s easy to start with, even for newbies.
- Flexibility: It’s used for both front-end and back-end tasks.
- Extensive Ecosystem: It has a big library of tools like React and Angular.
- Direct Browser Execution: You can test and run code right in the browser.
JavaScript Code Sample
Here’s a simple JavaScript example:
let message = "Hello, World!";
function showMessage() {
alert(message);
}
showMessage();
This code shows how JavaScript lets you define a variable without saying what type it is. It’s a basic example of JavaScript’s flexibility.
What is TypeScript?
TypeScript is made by Microsoft and is a super-set of JavaScript. It adds more features and strong typing to JavaScript. It’s great for big projects because it has strong type-checking and many tools.
Key Features
TypeScript has many features that make it better than regular JavaScript. Some of these key features are:
- Static Typing: This is what makes TypeScript special, helping catch bugs early.
- Interfaces and Generics: These make the code more efficient and reusable.
- Improved IDE Support: It offers great autocompletion, navigation, and refactoring tools, making work easier.
- Compatibility with JavaScript: Any JavaScript code that works is also fine in TypeScript.
Advantages of TypeScript
Using TypeScript has many benefits for big projects. Here’s why it’s so valuable:
- Enhanced Code Quality: It makes the code easier to read and keep up with.
- Quick Identification of Errors: Checking for errors before running the code cuts down on bugs.
- Better Collaboration: With clear types, working together on code is easier for everyone.
- Scalability: It’s perfect for big projects because of its clear structure and code.
TypeScript Code Sample
Let’s look at a TypeScript code example to see how it helps with type annotations and error checking:
// TypeScript code example
function greet(name: string): string {
return "Hello, " + name;
}
let user: string = "Alice";
console.log(greet(user));
This example shows how using type declarations helps handle variables correctly. It cuts down on runtime errors and makes the code more reliable.
JavaScript vs TypeScript: Typing Discipline
In the world of JavaScript vs TypeScript typing, knowing the difference between dynamic typing vs static typing is key for developers. They need to pick the right language for their projects. JavaScript and TypeScript have different typing systems that meet various development needs.
Dynamic Typing in JavaScript
JavaScript uses dynamic typing, which means you don’t have to say what type a variable is. This makes coding fast and easy, especially for small projects or prototypes. But, it can lead to errors that show up only when the code runs, which is a problem in big projects.
Static Typing in TypeScript
TypeScript, on the other hand, is all about static typing. You tell the type of a variable when you write the code. This makes the code more reliable by finding errors before it runs. Developers working on big projects like this for its strong type-checking, which makes their code more predictable and stable.
To wrap up, let’s compare JavaScript vs TypeScript typing with this table:
Aspect | JavaScript | TypeScript |
---|---|---|
Typing Discipline | Dynamic Typing | Static Typing |
Error Detection | Runtime | Compile-time |
Project Suitability | Small Projects, Prototypes | Large-scale Applications |
Compilation Differences
When we talk about JavaScript and TypeScript, we see how they differ in compilation. These differences change how we develop, handle errors, and run our code.
JavaScript Compilation
JavaScript is unique because it doesn’t need compiling. It runs directly in web browsers and places like Node.js. This means developers can quickly write and test their code, which is great for fast development and trying out new ideas.
This quick execution is a big reason why JavaScript is so popular with web developers.
TypeScript Compilation
TypeScript, however, does require a compilation step. It turns TypeScript code into JavaScript before running it. This step is important because it adds features like static type checking. This helps catch errors before the code runs, making applications more reliable.
But, this compilation step also makes the process slower. Developers need to think about the benefits of better error checking versus the need for quick development. This is important for keeping the workflow efficient.
Aspect | JavaScript | TypeScript |
---|---|---|
Compilation | None (interpreted) | Required (compiled to JavaScript) |
Error Detection | Runtime | Compile-time and Runtime |
Execution Speed | Fast due to immediate execution | Introduces compile-time overhead |
Development Experience | Rapid prototyping | Enhanced reliability and type safety |
Error Handling
Error handling is key in both JavaScript and TypeScript programming. How these languages handle errors can greatly affect how well a project works and the user’s experience.
Runtime Errors in JavaScript
JavaScript finds errors while the code is running. This is because it’s an interpreted language. So, errors are often found only when the code is executed. This makes debugging harder and requires a lot of testing to find and fix problems.
- Errors are caught only during execution.
- Increased debugging difficulty due to absence of pre-runtime checks.
- Potential for more bugs in production environments.
Compile-time Errors in TypeScript
TypeScript, on the other hand, checks for errors before the code runs. Being a statically-typed language, it spots errors during compilation. This means fewer bugs when the code is executed.
Here are the benefits of TypeScript’s error handling:
- Early detection of errors before code runs.
- Less time and effort in debugging.
- Less bugs in production thanks to early error catching.
Let’s compare how JavaScript and TypeScript handle errors:
Aspect | JavaScript Runtime Errors | TypeScript Error Handling |
---|---|---|
Detection | During code execution | During code compilation |
Debugging | More challenging due to post-execution identification | Easier due to pre-execution identification |
Bug Presence | Potentially higher in production | Lower due to early error detection |
Knowing these differences helps us see the pros and cons of JavaScript and TypeScript in handling errors. This knowledge lets developers pick the best tool for their projects. It ensures apps are more reliable and have fewer errors.
Development Experience & Tools
Looking into JavaScript and TypeScript development, we see big differences. These come from the special tools each language has. Let’s dive into these differences.
IDE Support
Integrated Development Environment (IDE) support is key in today’s development world. TypeScript gets a big boost from IDEs like Visual Studio Code. These offer auto-completion and real-time type checking. This makes coding faster and cuts down on mistakes.
JavaScript tools are also strong but don’t give the same quick feedback and structure help as TypeScript.
Refactoring Capabilities
Refactoring keeps code clean and efficient. TypeScript’s static typing makes refactoring safer and more predictable. It supports renaming variables and reorganizing code while keeping type safety in check.
JavaScript tools are powerful but need more careful, manual refactoring. This is because JavaScript is more dynamic.
Collaborative Development
How teams work together can be affected by the language and tools they use. TypeScript makes teamwork better by offering a structured, type-safe environment. This helps in understanding code and bringing new team members up to speed fast.
Its static types keep projects consistent and reliable. JavaScript is better for quick prototyping and small teams or solo projects. It’s flexible and has less strict entry requirements.
Use Cases for JavaScript vs TypeScript
Choosing between JavaScript and TypeScript depends on your project’s needs. Each language has its strengths. Knowing when to use each can help developers make better choices.
When to Choose JavaScript
JavaScript is great for small to medium projects. It’s flexible and perfect for quick prototyping. This makes it ideal for startups and projects with tight deadlines.
JavaScript also lets you run code directly without extra steps. This makes development faster, which is key in fast-paced projects. Plus, it has a huge library of tools like React, Angular, and Vue.
When to Choose TypeScript
TypeScript shines in large projects needing complexity and maintainability. It offers static typing for extra type safety, lowering runtime errors. This is great for big projects or team work where keeping code consistent and preventing errors is key.
TypeScript also has strong IDE support and better refactoring tools. These features make development smoother and more efficient.
In summary, choosing between JavaScript and TypeScript depends on your project’s size and complexity. JavaScript is good for small projects because it’s easy to use and flexible. TypeScript is better for big projects because it’s structured and has advanced tools.
Conclusion
As we conclude our look at JavaScript vs TypeScript, we see both languages have their strengths. The JavaScript programming language is great for quick projects and beginners. It’s flexible and easy to use, thanks to its dynamic typing.
TypeScript, however, shines with its static typing and better tool support. It’s ideal for big projects because it helps find errors early. This makes TypeScript a top choice for complex and growing projects.
Knowing the strengths and weaknesses of each language helps us pick the best tool for our projects. By understanding JavaScript and TypeScript, we can improve our work’s efficiency, reliability, and growth. Choosing between them depends on the project’s needs, the team’s skills, and the goals for the future.
FAQ
What are the key differences between JavaScript and TypeScript?
JavaScript and TypeScript differ mainly in their typing systems. TypeScript uses static typing, which means you must define types for variables. This helps catch errors before running the code. JavaScript, on the other hand, uses dynamic typing, letting you change a variable’s type later. TypeScript also gets turned into JavaScript before running, while JavaScript runs directly.
What are the core features of JavaScript?
JavaScript is known for being interpreted, having dynamic typing, and supporting event-driven and asynchronous programming. It’s also supported by many web browsers and frameworks. These features help make web pages interactive and responsive.
Why should I use JavaScript for my project?
JavaScript is great for small to medium projects because it’s simple and flexible. It runs directly in web browsers, making it quick to deploy. It also has a big library of tools that help with making and testing projects fast.
Can you provide a JavaScript code sample?
Sure! Here’s a simple JavaScript code:
let message = "Hello, World!";
console.log(message);
This code shows how JavaScript doesn’t need type definitions for variables. The `message` variable is set to a string without declaring its type.
What are the key features of TypeScript?
TypeScript is known for its strong typing system, including generics and interfaces. It also has better support in development environments and checks for errors before running the code. These features make large projects clearer, easier to maintain, and more predictable.
What are the advantages of using TypeScript?
TypeScript is great for big projects because it improves code quality and makes development tools better. Its static typing helps prevent errors and makes coding safer. It also helps teams work together more smoothly.
Can you provide a TypeScript code sample?
Of course! Here’s a basic TypeScript code:
let message: string = "Hello, World!";
console.log(message);
This code shows TypeScript’s static typing by defining `message` as a string explicitly.
What is dynamic typing in JavaScript?
Dynamic typing in JavaScript means the type of a variable is decided at runtime. This lets developers change a variable’s type anytime. But, it can lead to errors if types don’t match.
What is static typing in TypeScript?
In TypeScript, static typing means you must define types for variables before running the code. This catches errors early and makes the code better. It helps avoid runtime errors and makes coding safer.
How does JavaScript handle compilation?
JavaScript doesn’t need a separate compilation step. It runs directly in web browsers or Node.js. This makes developing projects quick and efficient.
How does TypeScript handle compilation?
TypeScript needs to be turned into JavaScript before running. This process checks for type errors and makes sure the code meets strict standards. It then turns into reliable JavaScript that works in any browser or environment.
How does JavaScript handle errors at runtime?
JavaScript finds errors while running, which can make fixing them hard. Developers use thorough testing to find and fix problems after the code runs.
How does TypeScript handle errors?
TypeScript finds errors before running the code, during compile-time. This early check helps developers spot and fix issues quickly. It makes the code more reliable and prevents common runtime errors.
What development tools and features support JavaScript and TypeScript?
JavaScript has many libraries, frameworks, and tools for quick development and deployment. TypeScript, however, has better support in IDEs, auto-completion, refactoring tools, and collaboration features because of its static typing.
When should I choose JavaScript over TypeScript?
Choose JavaScript for small projects or quick prototyping. It’s flexible and quick to deploy. Its dynamic nature is great for changing requirements and fast testing.
When should I choose TypeScript over JavaScript?
Pick TypeScript for big, complex projects needing maintainability and type safety. Its static typing and better tools support strong development practices. It’s especially good for working with teams.
Future App Studios is an award-winning software development & outsourcing company. Our team of experts is ready to craft the solution your company needs.