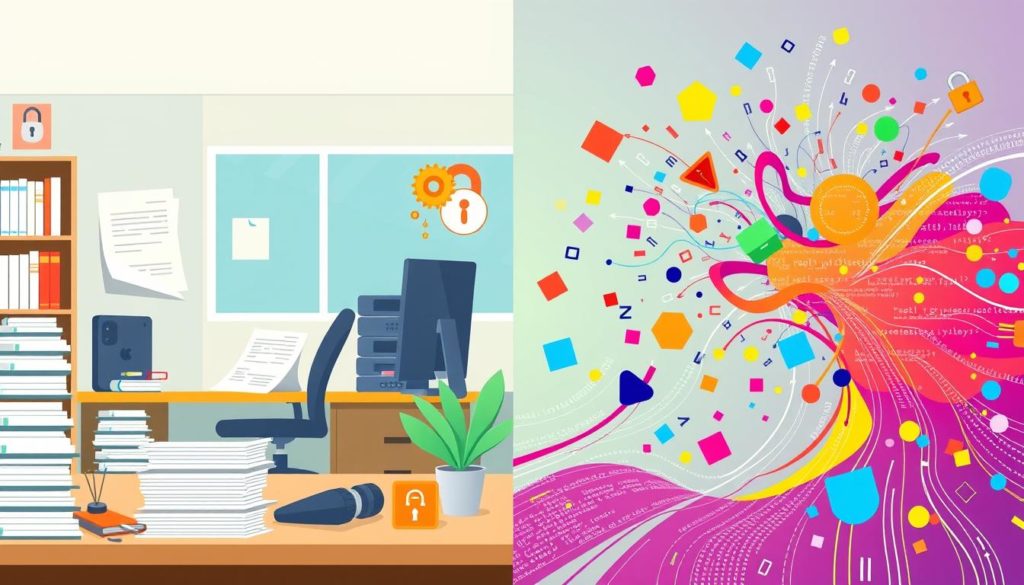
Did you know teams focusing on user experience can see up to 40% better performance? This shows how important the right programming languages are. Choosing between static and dynamic typing affects how we handle variables and errors.
Programming typing is about sorting data and variables into types like integers or strings. This sorting is key because it shapes how data is handled and instructions are processed. The debate over static vs dynamic typing revolves around early error detection versus flexibility.
Static typing languages, like Java and C, need variables to be typed at compile-time. This helps catch errors early, making code safer and faster. It’s a way to ensure code works right before it’s even run.
Dynamically typed languages, such as Python and Ruby, assign types at runtime. This makes coding easier but can lead to more errors. JavaScript’s TypeScript is a mix, aiming to catch errors early while keeping coding flexible.
Exploring static vs dynamic typing shows how they affect error handling and development speed. Finding the right balance is crucial for team success and software performance. For more on the differences, check out this resource.
Key Takeaways
- Typing in programming involves categorizing data and variables based on their data types.
- Static typing catches common mistakes during compilation, ensuring early error detection.
- Dynamically typed languages offer flexibility but can be more error-prone during execution.
- Examples of statically typed languages include Java, C, and C++, while Python and JavaScript are dynamically typed.
- JavaScript’s creation of TypeScript shows an effort to combine static typing benefits with dynamic language flexibility.
Introduction to Typing in Programming Languages
Learning about typing in programming is key for all developers. It helps manage data types and ensure variables are categorized correctly. This is crucial for programming.
What is Typing?
Typing in programming means defining data types for variables like integers and strings. It keeps data safe and ensures operations work right. By categorizing variables, we manage memory better and make programs run smoothly.
Many modern languages use type inference. This means they can guess the variable type without needing to be told. This makes coding easier.
Importance of Typing for Error Handling
Typing is vital for handling errors well in software development. It helps catch mistakes early, especially in statically typed languages. These languages check types before running the code, avoiding many errors.
Good typing practices lead to more reliable code. It also makes debugging easier by showing what data types are expected.
Static vs Dynamic Typing Overview
Static and dynamic typing are two main ways to handle variable types. Static typing checks types at compile time and keeps them the same. This makes code safer and catches errors early.
Dynamic typing, on the other hand, checks types at runtime. Languages like Python and JavaScript let types change as values do. This makes coding faster but can lead to runtime errors.
Knowing the differences between static and dynamic typing helps developers choose the right approach for their projects. Each has its own benefits and challenges.
What is Static Typing?
Static typing is a way programming languages check types before running the code. It makes developers state the types of their variables upfront. This helps find errors early and makes the code run faster.
Compile-Time Type Checking
Compile-time type checking is key in static typing. It spots type errors before the program starts. This makes the code more reliable and stable.
Strong Typing and Type Safety
Strong typing means variables must match their declared types. This boosts type safety and cuts down on runtime errors. Languages like C, Java, and Rust follow these rules closely.
This strict typing makes code easier to read and maintain. It also helps developers use tools like code completion and refactoring more effectively.
Examples of Statically Typed Languages
Many well-known programming languages are statically typed. Here are a few:
- C and C++: Great for high-performance tasks and low-level operations
- Java: Ideal for big business projects, thanks to its strong type checking
- Rust: Focuses on safety and concurrency, with strong compile-time checks
These languages are often picked for big projects because they find errors early and run fast. They also make code easier to understand and maintain over time.
What is Dynamic Typing?
Dynamic typing means the type of a variable is figured out when the code runs, not before. This makes coding easier because you don’t have to say what type of data a variable will hold. Languages like Python, JavaScript, PHP, and Ruby use dynamic typing to make coding simpler.
But, dynamic typing can lead to more runtime errors. This is because the type of a variable is checked only when the code runs. If the types don’t match, unexpected things can happen. Still, many developers like dynamic typing for its ease and flexibility, especially in interpreted languages.
Big names in programming, like Robert Martin and Bruce Eckel, have moved from statically typed languages to dynamically typed ones. They prefer languages like Python for its ease and speed. This shows a trend towards using dynamic typing for some projects.
Dynamic typing might not catch errors as early as static typing. But, it’s great for quick prototyping and development. For example, Smalltalk’s self-testing code shows that bugs can be found through testing, even with dynamic typing.
Here’s a comparison of dynamic and static typing:
Feature | Dynamic Typing | Static Typing |
---|---|---|
Type Determination | At runtime | At compile time |
Type Flexibility | High | Low |
Error Detection | During execution | Earlier in development |
Verbose Type Declarations | Not required | Required |
Code Readability | Variable | Consistent |
Choosing dynamic typing lets developers work more flexibly and efficiently. They should be aware of the chance for runtime errors. Using interpreted languages with dynamic typing makes programming smoother, especially for quick projects.
Static vs Dynamic Typing
The debate between static and dynamic typing is about flexibility versus safety. This choice affects how fast and reliable a project can be. Statically typed languages, like C++ and Java, check types before running the code. This means variables can’t change types later, making the code safer and faster.
This approach is great for big projects where things need to work smoothly. It helps find errors early.
Flexibility in Variable Types
Dynamic typing, found in languages like Python and JavaScript, offers more flexibility. You can change a variable’s type easily without saying what it is. For example, in Groovy, you can just write num = 5
without saying it’s an integer.
This makes coding faster but might lead to errors when the code runs. It’s perfect for quick projects or scripts.
Runtime Type Checking
Dynamic languages check types when the code runs, not before. This means errors might show up when you least expect them. It’s different from static typing, where errors are caught early.
Even with this risk, dynamic typing is great for fast development. It’s perfect for quick prototypes or scripts.
Examples of Dynamically Typed Languages
Python, JavaScript, and Groovy are examples of dynamic typing. They’re popular for web development and data science because they’re easy to use. The choice between static and dynamic typing depends on what your project needs.
FAQ
What is Typing in Programming Languages?
Typing in programming means sorting data and variables into types like integers, strings, or booleans. This sorting is key because it tells a language how to handle data.
Why is Typing Important for Error Handling?
Typing is key for error handling because it keeps data and code reliable. Languages that check types before running the code can spot errors early.
Can you give an Overview of Static vs Dynamic Typing?
Static typing checks types before running the code. This means a variable’s type is set before the program starts. Dynamic typing, however, sets types while the code is running. This makes it flexible but can lead to errors.
Static typing is seen in languages like Java and C++. Dynamic typing is found in languages like Python and JavaScript.
What is Static Typing?
Static typing is when a program checks types before it runs. This makes sure variables have specific types, making the code safer and catching errors early.
What are Compile-Time Type Checking and its Benefits?
Compile-time type checking happens when a program checks variable types before it runs. This makes the code safer by catching errors early, reducing runtime errors.
What is Strong Typing, and How Does it Relate to Type Safety?
Strong typing is a strict version of static typing. It makes sure types match exactly, reducing errors and making the code safer.
What are Some Examples of Statically Typed Languages?
Languages like C, C++, Java, and Rust are statically typed. They require specific types for variables, making the code safer and catching errors early.
What is Dynamic Typing?
Dynamic typing lets variable types change while the code runs. This makes programming easier but can lead to errors if not managed well.
What are the Potential Downsides of Dynamic Typing?
Dynamic typing can lead to errors since types are checked only when the code runs. This can cause unexpected behavior if not managed right.
How does Dynamic Typing Offer Flexibility in Variable Types?
Dynamic typing lets variable types change during execution. This makes development easier, especially in fast-changing or experimental projects.
What is Runtime Type Checking?
Runtime type checking happens when a variable’s type is checked while the code runs. This is common in dynamic languages and can cause errors if not managed well.
Can you Give Examples of Dynamically Typed Languages?
Languages like Python, JavaScript, PHP, and Ruby are dynamically typed. They assign types while the code runs, offering flexibility but needing careful handling to avoid errors.
What are the Key Differences between Static and Dynamic Typing?
Static typing is about fixed types checked before running the code, leading to early error detection and better performance. Dynamic typing lets types change during execution, offering flexibility but with a higher risk of errors.
Future App Studios is an award-winning software development & outsourcing company. Our team of experts is ready to craft the solution your company needs.