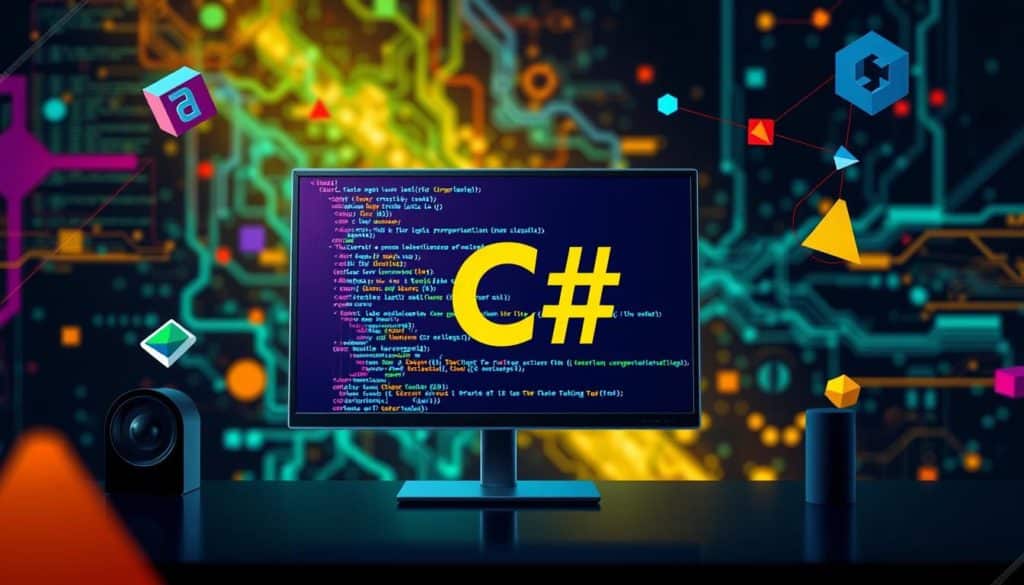
Did you know C# is the #4 programming language, just behind Java and JavaScript? It’s a great choice for beginners. Learning C# can open up exciting opportunities.
C# was created by Microsoft’s Anders Hejlsberg in 2000. It was first called COOL, then renamed to C#. It works well with the .NET framework. This makes it perfect for creating desktop apps, web apps, and games.
Major programs like Microsoft Visual Studio and Paint.NET use C#. This C# tutorial is for beginners. You don’t need any coding experience. We’ll cover the basics and show you why C# is great for developers.
We’ll teach you how to set up your environment and write your first C# program. This hands-on learning will show you how easy and powerful C# is.
C# is a top-ten language in the TIOBE Index. It’s easy to learn and has a strong community. This makes it a great choice for beginners and experts.
For more on C#, check out this comprehensive beginner’s guide to C#. It has lots of examples and explanations to help you learn.
Key Takeaways
- C# ranked #4 on the PYPL Popularity of Programming Language Index as of November 2022.
- Developed by Microsoft’s Anders Hejlsberg in 2000.
- Originally called COOL (C-like Object Oriented Language).
- Widely used for desktop applications, web apps, and game development.
- Integrated with the .NET framework for scalable and high-performance applications.
Introduction to C#
C# is a modern programming language from Microsoft, part of the .NET initiative. It’s known for its robust framework, making it a top pick for many projects. We’ll explore its history and key features in this section.
History of C#
C# was introduced in the early 2000s by Microsoft as part of .NET. Anders Hejlsberg and his team created it. It was designed to offer a modern, object-oriented alternative to Java on Windows.
Since then, C# has become a favorite among developers worldwide. It’s used in countless applications, showing its versatility and strength.
Overview of C#
C# is a type-safe, object-oriented language. It’s easy to learn for beginners and powerful for experts. Its syntax is similar to C, C++, JavaScript, and Java, making it familiar to many.
C# supports asynchronous programming, pattern matching, and LINQ. This makes coding efficient and easy to read. Its memory management and large library also add to its appeal.
C# excels in web, desktop apps, and game development. It’s great for working with Microsoft technologies. With tools like Xamarin and .NET MAUI, you can build cross-platform apps.
C# offers strong memory management and a wide range of tutorials. It’s perfect for both simple and complex projects. Learning C# basics will help you unlock its full potential.
Why Learn C#?
C# is a strong and flexible programming language. It’s widely used and has a big community of developers. Microsoft supports it, making sure it keeps getting better. It’s one of the top five programming languages, known for its power and versatility.
Versatility and Power
C# can be used for many things. You can make web sites, mobile apps, or even games. It works well with the .NET framework, which is loved by many developers.
Learning C# means you can work on different platforms. .NET Core makes it work on Linux and Mac too. This makes C# very flexible and popular.
Career Opportunities
Learning C# can lead to great jobs. It’s used in big companies for complex projects. Microsoft and others use it a lot.
It’s also great for game development and web sites. Knowing C# makes you valuable in the job market.
Here is a comparison chart illustrating the areas where C# excels:
Application Area | Description | Examples |
---|---|---|
Web Development | Using ASP.NET for building dynamic web applications | eCommerce sites, Content Management Systems |
Mobile Development | Creating cross-platform mobile apps via Xamarin | Business apps, Mobile games |
Game Development | Developing high-performance games with Unity | 3D and 2D games, Virtual reality experiences |
Enterprise Applications | Building large-scale, reliable enterprise systems | CRM systems, ERP software |
In conclusion, C# is a great choice for developers. It’s versatile, powerful, and offers many job opportunities.
Setting Up Your Development Environment
Creating a strong C# development environment is key for good programming. You need to pick the right Integrated Development Environment (IDE) and C# Compiler. C# is a compiled language, which means it turns into machine code quickly. This results in standalone executable files.
Recent surveys show that 50% of tutorials suggest using Visual Studio for Windows. Another 25% recommend Visual Studio Code with C# DevKit. The remaining 25% suggest other editors that need the latest .NET SDK. Most tutorials (75%) start with a console application, and all assume you’ve done the “Hello World” lesson first.
- Download and Install Visual Studio Community Version: This version is free from Microsoft and great for learning. Windows already has the .NET Framework, making setup easy.
- Install the .NET SDK: If you’re using an editor other than Visual Studio, you’ll need to download the latest .NET SDK. The .NET Framework supports C# and has two main parts: Common Language Runtime (CLR) and Framework Class Library (FCL).
- Configure Your IDE: Make sure your IDE targets .NET 5 or higher. This is the most recommended framework for tutorials.
We’ve made a table to help you understand recent tutorials better:
C# Setup Component | Percentage of Tutorials |
---|---|
Visual Studio for Windows | 50% |
Visual Studio Code with C# DevKit | 25% |
Different Editor with .NET SDK | 25% |
Introduction with Console Application | 75% |
‘Hello World’ Lesson Before Other Tutorials | 100% |
Specific Lessons Before Next Tutorial | 75% |
.NET CLI Commands in Instructions | 3 commands |
By following these steps and understanding common practices, you’re ready to create a productive C# development environment. Whether you choose Visual Studio or another editor, having the right C# setup is crucial for successful programming.
Basics of C# Syntax
Learning the basics of C# syntax is key for anyone starting with C# coding. C# makes coding clear and easy to manage. It focuses on variables, data types, operators, and conditional statements. Let’s dive into these basics.
Variables and Data Types
In C# syntax, variables hold data, and data types tell what kind of data they hold. Variables must start with a letter and can’t have special symbols. Here’s how to declare a variable:
int length;
float width;
string rectangleName;
Some common data types in C# are:
- int: Whole numbers
- float: Floating-point numbers
- string: A sequence of characters
Operators
Operators in C# do operations on variables and values. They’re vital for C# coding and fall into several types:
- Arithmetic Operators: +, -, *, /, %
- Comparison Operators: ==, !=, >, =,
- Logical Operators: &&, ||, !
Here’s an example of operators in use:
int a = 10;
int b = 20;
int sum = a + b; // Adds a and b
bool isEqual = (a == b); // Checks if a is equal to b
Operator | Function |
---|---|
+ | Addition |
– | Subtraction |
* | Multiplication |
/ | Division |
% | Modulus |
Conditional Statements
Conditional statements control program flow based on conditions. They’re key to C# syntax, making programs dynamic and responsive. The main conditional statements in C# are:
- if statement
- else statement
- else if statement
- switch statement
Here’s an example of an if-else statement:
int number = 10;
if (number > 0) {
Console.WriteLine("The number is positive.");
} else {
Console.WriteLine("The number is negative.");
}
Knowing variables, data types, operators, and conditional statements is crucial. It’s the first step to mastering C# syntax and writing effective code.
What is C#
C# is a programming language that supports many ways of coding, like structured, object-oriented, and functional programming. It was created by Anders Hejlsberg and first came out in 2000. The latest version, C# 12.0, was released in 2023 with the .NET 8.0 framework. It’s known for being modern and strong, perfect for making software parts that work together well.
Object-Oriented Programming
C# is all about object-oriented programming (OOP). This way of coding helps make code reusable and organized. It uses classes, inheritance, polymorphism, and encapsulation to build strong systems. C# makes designing software easier and more efficient.
For more details on C#’s history and how it works, check out the full C# language description.
Type Safety
Type safety in C# makes the language reliable and cuts down on errors. It makes sure variables only hold the right type of data. This helps find mistakes early on. C# supports different types of data, making it better at managing memory and working smoothly.
Here’s a quick look at what makes C#’s OOP and type safety great:
Principle | Description | Benefits |
---|---|---|
Classes | Blueprints for creating objects | Reuse code, create modular designs |
Inheritance | Derive new classes from existing ones | Promote code reusability and extend functionality |
Polymorphism | Ability of different classes to be treated as instances of the same class | Simplify code management |
Encapsulation | Bundling data with methods that operate on the data | Hide internal state, safeguard data integrity |
Type Safety | Ensuring variables are assigned correctly | Minimize runtime errors, enhance stability |
These key ideas of object-oriented programming C# and type safety in C# show why C# is a top choice for developers. It helps build apps that are big, efficient, and reliable.
Exploring C# Features
C# is known for its wide range of features. These features help developers work more efficiently and build strong applications. We will look at two key areas: asynchronous programming and reflection in C#.
Asynchronous Programming
Asynchronous programming in C# lets developers run long tasks without slowing down the main program. This is great for apps that need to be quick and responsive. It uses the async and await keywords.
For example, when doing network tasks or database queries, it keeps the app’s interface smooth and easy to use.
Reflection in C#
Reflection in C# is a strong tool for looking into and changing code details at runtime. It lets developers check and change methods, properties, and more. This is super useful for automated tests, data saving and loading, and more.
Common Use Cases for C#
C# is a versatile programming language used in many areas. It has made a big impact on web and mobile development. It also works well with Unity in game development. This makes C# a great choice for creating strong solutions for different needs.
Web and Mobile Development
In web development, C# plays a key role. It works well with ASP.NET Core to make fast, secure C# web applications. Frameworks like Blazor help developers build modern web apps. C# also supports mobile app development for Android and iOS with Xamarin Forms and .NET MAUI.
There are many libraries and packages for C# development. Over 300,000 Nuget packages are available for enterprise apps. This shows how versatile C# is, from simple websites to complex apps.
Game Development
In game development, C# is the top choice, thanks to Unity. Unity, built with C#, is a leading game engine. It lets developers make engaging 2D and 3D games. Unity is loved for its ease of use and strong scripting.
Unity has over a billion users and is trusted by 1.5 million developers. It supports high-performance games and cross-platform development. This lets developers reach players on many devices and consoles.
C# also has features like asynchronous streams and pattern matching. These help make games run smoothly and efficiently. This makes C# a top pick for creating high-quality games.
Development Area | Framework/Tool | Key Features |
---|---|---|
Web Applications | ASP.NET Core | Scalable, secure, high performance; supports Blazor for modern SPAs |
Mobile Applications | Xamarin Forms and .NET MAUI | Cross-platform development for Android and iOS |
Game Development | Unity | 2D and 3D game development, high performance, cross-platform support |
In conclusion, C# is a solid base for web and game development. It works with powerful tools like ASP.NET Core and Unity. This lets developers create engaging experiences on many platforms.
Conclusion
C# is a powerful programming language that plays a big role in software development today. It was released in 2000 and has become a versatile and strong language. It’s well-suited for the .NET platform.
As we explored, C# has many features. These include object-oriented principles, the ability to work on different platforms, and a big community of users. All these make C# popular and useful.
C# can be used for many types of applications. This includes desktop and mobile apps, web services, and even games. This variety leads to many job opportunities for C# developers. These jobs are in demand and can be very rewarding.
While C# has some limitations, like extra overhead and a focus on Microsoft platforms, its benefits are greater. Learning C# can lead to a bright future in software development.
Mastering C# can help you create many different, high-quality applications. It also helps you grow professionally. C# keeps getting better with updates like C# 11 and a wide range of tools and resources.
Looking back, C# meets today’s tech needs and encourages tomorrow’s innovations. It’s clear that C# is a key part of the coding world.
FAQ
What is C#?
C# is a programming language made by Microsoft. It’s part of the .NET initiative. It’s used for making many types of applications, from desktop software to web services.
Why should I learn C#?
Learning C# can lead to many career opportunities. It’s used in web, mobile, and game development. It helps you make high-performance applications and improve your coding skills.
How do I set up my development environment for C#?
To start with C#, you need an Integrated Development Environment (IDE) like Visual Studio. You also need a C# compiler. Setting these up on your computer makes coding in C# easy.
What are the basics of C# syntax?
C# basics include variables, data types, operators, and conditional statements. These help you write clear and effective C# programs.
What is Object-Oriented Programming in C#?
Object-Oriented Programming (OOP) in C# helps make code modular and reusable. It uses classes, inheritance, polymorphism, and encapsulation. This makes your code easier to maintain and grow.
What does type safety in C# mean?
Type safety in C# means strict data type rules. This reduces errors and makes code more reliable. It ensures data operations are correct, improving program stability.
What are asynchronous programming and reflection in C#?
Asynchronous programming in C# makes tasks run without blocking the main thread. Reflection lets you inspect and change code at runtime. This adds dynamic features to your programs.
How is C# used in web and mobile development?
C# is used in web development with ASP.NET and in mobile with Xamarin. Its cross-platform abilities ensure apps work well on different systems.
What role does C# play in game development?
C# is key in game development, especially with Unity. It helps create fast, high-quality games. Its object-oriented features and libraries are very useful.
Future App Studios is an award-winning software development & outsourcing company. Our team of experts is ready to craft the solution your company needs.