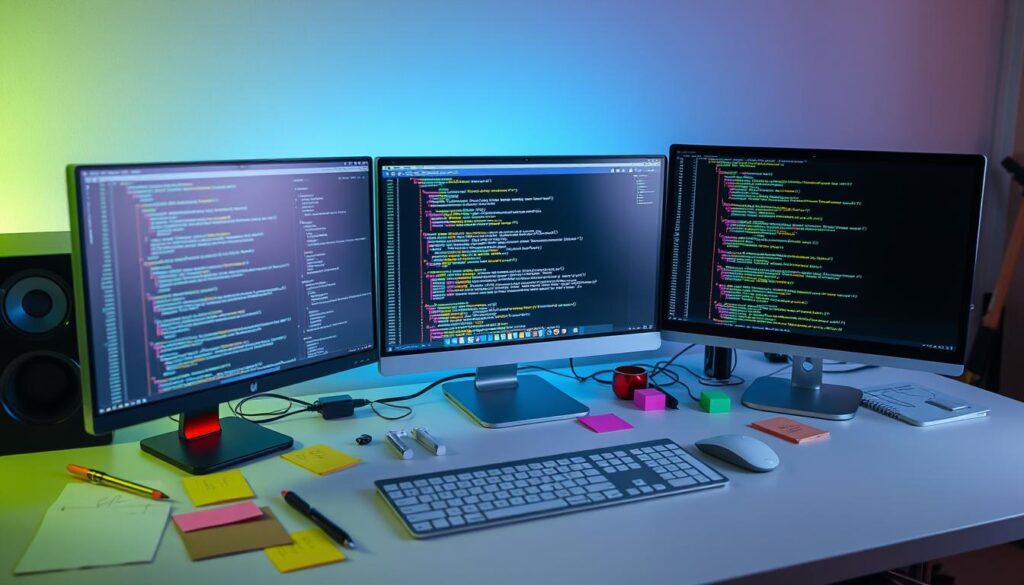
Did you know debugging takes up 40% of coding time? This shows how important and time-consuming it is in software development. Learning to debug well is key to coding efficiently and keeping programs running smoothly.
In software development, bugs are a normal part of the job. Even experienced developers spend more time fixing bugs than writing new code. This process helps us improve our coding skills and solve unexpected problems. By getting better at debugging, we turn these challenges into chances to learn.
Good debugging needs a plan. It’s about understanding error signs, finding the problem, and fixing it. Tools like breakpoints and debugging tools are essential. Planning our code helps avoid many bugs, saving us time and effort.
Using methods like hypothesis-driven debugging and automated testing helps fix bugs fast. These strategies, along with checking our code carefully, help us find and fix bugs well.
Key Takeaways
- Debugging takes up 40% of coding time, showing its key role in software development.
- Bugs are a normal part of coding, no matter how skilled you are.
- Effective debugging includes techniques like breakpoints, backtracking, and rubber duck debugging.
- Planning and understanding our code beforehand can prevent many bugs.
- Strategies like hypothesis-driven debugging and automated testing are crucial for maintaining software quality.
Understanding the Importance of Debugging in Coding
Debugging has been a key part of software development since the 1960s. It’s different from coding because it needs a careful eye to find and fix problems. Knowing why debugging takes more time than coding helps us understand the software-making process better.
Many things make debugging take a lot of time. For example, syntax errors are easy to spot with code editors. But semantic errors, like y = x / 2 * math.pi, can lead to wrong results and need more effort to solve. Logic errors, like programs ending loops too soon or giving wrong results in if statements, also add to the debugging time.
There are ways to make debugging easier. For instance, making small parts of the program and testing them often helps find bugs. Going back from a problem to find where it started is another method. Tools for debugging in different places also help solve issues.
Debugging in the cloud is especially tricky. It needs special tools because of the differences in settings. Amazon Web Services (AWS) has tools for Eclipse to help with Java coding and debugging. AWS X-Ray helps analyze how well applications work, making it easier to find and fix problems.
Table 1 below shows common programming errors and how to fix them:
Type of Error | Description | Debugging Technique |
---|---|---|
Build and Compile Time Errors | Occur during development, preventing application functions. | Syntax checks, Editor highlighting |
Runtime Errors | Occur during application execution, often dependent on user input or environment. | Try-catch blocks, Memory management |
Logic Errors | Occur after the program runs, leading to incorrect outcomes. | Step debugging, Backtracking |
Developers must see the value in debugging. It may take a lot of time, but it helps make software better. As developers get better at debugging, they work more efficiently. This makes sure the software is stable and safe, showing why debugging takes more time than coding.
Top Debugging Techniques Developers Should Know
Debugging is key for developers to make sure their code works well. Let’s explore some top debugging techniques and tools. These can greatly improve how you solve problems.
Most modern IDEs have built-in debuggers. These let developers set breakpoints, check variables, and go through code step by step. It’s important to set breakpoints wisely. They pause the program at certain points, making it easier to find issues.
Using the binary search method is also helpful. It finds bugs by splitting the code into parts and narrowing down where the problem is. This method is great for complex systems.
Log analysis is crucial for debugging. It shows how the program runs and what values variables have. Tools like Chrome DevTools for front-end work or the Visual Studio Debugger for C# can make debugging easier.
“Rubber Duck Debugging” is another strategy. It involves explaining problems out loud or to an object. It might sound strange, but it’s very effective and helps you understand your code better.
Also, research shows developers spend about 50% of their time fixing errors. Using good techniques and tools can cut down this time a lot.
Here’s a table of some top debugging tools and what they’re good for:
Debugging Tool | Specialization |
---|---|
Chrome DevTools | Debugging of HTML/CSS and JavaScript |
Visual Studio Debugger | Rich debugging for C# development |
Postman | API Testing and Debugging |
SonarQube | Static Code Analysis |
Fiddler | Web Requests and Responses Analysis |
In summary, using a mix of these techniques and tools can really improve how we debug. By using systematic methods like binary search and powerful tools, we can better find and fix bugs in our code.
Best Practices for Effective Error Diagnosis
Effective error diagnosis starts with understanding the error symptoms. It’s about finding the root cause step by step. We use tools like Visual Studio Code and Chrome DevTools to do this.
First, we analyze error symptoms with various tools. IDE debuggers and log analysis tools are our favorites. After understanding the problem, we find the root cause by reviewing the code and using static analysis.
Then, we use strategies to make sure we catch all issues. Profiling tools like DynaTrace help us see performance in real-time. We break down problems, reproduce them, and test solutions with print statements and breakpoints.
Collaboration is key in our error diagnosis. Working together helps us find insights we might miss alone. This way, we use everyone’s knowledge to solve problems.
Documentation is crucial during debugging. We document error descriptions, steps to reproduce, and changes made. This helps solve current problems and prepares for future ones.
Testing fixes is essential. We make sure our solutions work without causing new problems. This is a big part of our systematic approach.
Common Software Bugs | Diagnosis Strategy | Resolution Paths | Associated Tools |
---|---|---|---|
Logic errors | Using debugging tools | Fixing the root cause | Visual Studio Code |
Syntax errors | Reviewing the code | Testing the fix | Chrome DevTools |
Runtime errors | Implementing logging | Documenting the fix | DynaTrace, WinDbg |
Keeping up with new techniques and tools improves our debugging. Understanding error symptoms helps us fix bugs and ensure our software works well.
Advanced Troubleshooting Strategies
We’re diving into advanced troubleshooting strategies. We’re learning to tackle complex issues with ease. Post-Mortem Debugging is a key technique. It helps us understand why a program crashed.
Remote debugging is also important in today’s world. It lets us check apps on different servers. Tools like Chrome DevTools make this easier.
Breakdown of Developer Tasks | Key Focus Areas | Debugging vs. Testing |
---|---|---|
Debugging – 50% | Advanced Debugging Techniques | Testing: Prevents bugs before they occur |
Reproducing the Issue – 20% | Specialized Debugging Tools | Debugging: Reactive, addresses existing bugs |
Gathering Information – 30% | Basic Debugging Strategies |
Mastering Post-Mortem Debugging and remote debugging is key. We also need to use advanced tools for better performance. AI tools can help us solve complex problems remotely.
Code Optimization for Better Bug Fixing
The Importance of Writing Clean Code is huge in software development. Developers spend a lot of time on maintenance, not making new features. Up to 80% of their time goes to keeping things running smoothly.
Refactoring is key to fixing bugs. Experts say to refactor often to keep code easy to read and fix. This makes it easier to find and fix problems.
Using automated testing helps a lot with fixing bugs. Tests, especially unit tests, find bugs early. This makes it easier to fix them before they get worse.
Also, using dependency injection and aspect-oriented programming helps. These methods make code easier to test and fix. They let us add dependencies as needed, making code more modular.
Here are some stats on how well these methods work:
Optimization Technique | Efficiency Improvement |
---|---|
Refactoring | 30% reduction in post-release bugs |
Unit Testing | 40% decrease in critical bugs |
Code Reviews | 15% faster bug fixing |
Writing clear code is also key to avoiding bugs. Too complex or too simple code can lead to mistakes. Clean code makes programs better to debug and maintain.
In short, the Importance of Writing Clean Code is huge. Using methods like automated testing and refactoring leads to better software. It makes our work more efficient and our products better.
Learning from Each Debugging Session
Every debugging session is a chance to get better at solving problems. Keeping detailed records of the process helps us spot common mistakes. This way, we can learn from our mistakes and solve problems faster next time.
In schools, it’s important to see mistakes as chances to learn. Using a “Bug Museum” or “Bug Hall of Fame” can make learning fun. Giving students bug toys for solving problems can also make them excited to learn.
Using pseudocode can help find problems early, especially in big projects. Testing and debugging in parts helps catch bugs before they get worse. Paying attention to small details like spelling and punctuation is key. Tools like Visual Studio Code make debugging easier by offering built-in debuggers for many languages.
Learning from each session and working with others makes us better at solving problems. Taking a step back to see the bigger picture can also help solve tough issues. Seeing debugging as a chance to grow makes us better developers.
FAQ
Why is debugging considered an essential part of the software development lifecycle?
Debugging is key because it takes up a lot of time. Often, it’s 40% of the development time. It ensures the software works right and fixes unexpected problems.
What are some common debugging challenges developers face?
Developers struggle to find the exact problem in complex code. They also face challenges in fixing issues without creating new bugs. Finding a complete solution is another hurdle.
How can setting breakpoints help in the debugging process?
Breakpoints let developers pause the code at specific points. They can then check variable states and see how the program flows. This makes finding and fixing errors easier.
What is the binary search method in debugging?
The binary search method narrows down error sources by checking midpoints. It helps find the root cause by reducing the number of possibilities.
Can log analysis improve debugging efficiency?
Yes, log analysis is a great way to debug. It gives detailed insights into program execution. Developers can track down issues by analyzing logs to see where and why errors happen.
What are systematic steps for error diagnosis?
Systematic error diagnosis starts with understanding symptoms. Then, reproduce the problem, find the cause, fix it, and verify. Documenting the process helps improve future debugging.
How does post-mortem debugging work?
Post-mortem debugging analyzes software states after a crash. It’s useful for finding problems in deployed apps where real-time debugging isn’t possible.
What are the benefits of clean code in debugging?
Clean code has fewer bugs and is easier to understand. This makes debugging quicker and leads to better software quality.
How can we use automated testing to enhance debug efficiency?
Automated testing catches bugs early. This makes debugging more efficient by solving issues before they get complicated.
Why is it important to document the debugging process?
Documenting debugging helps track issues and solutions. It improves problem-solving skills and provides a reference for future sessions. It also helps developers grow.
Future App Studios is an award-winning software development & outsourcing company. Our team of experts is ready to craft the solution your company needs.