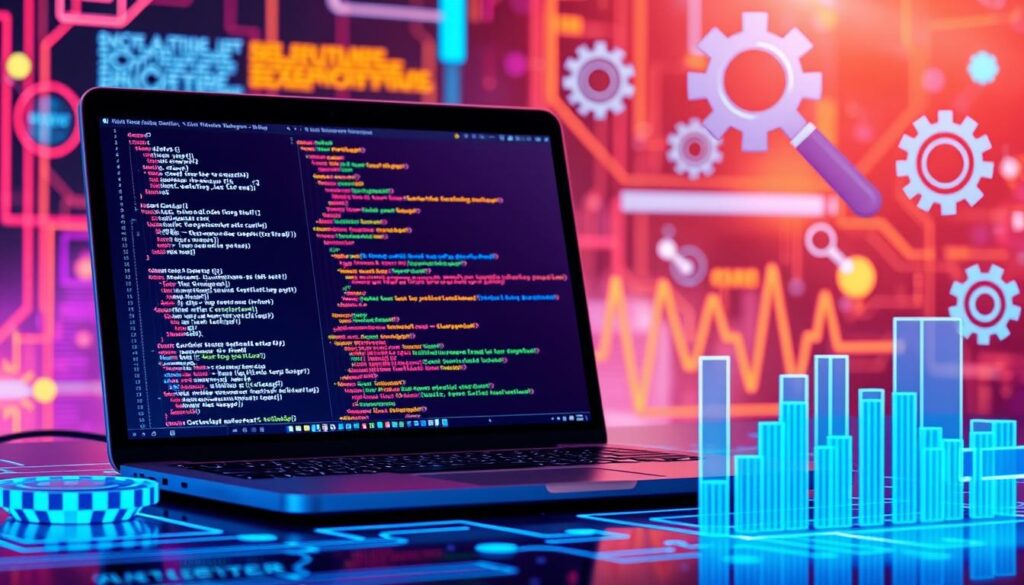
Did you know that using breakpoints can cut down debugging time? They help us focus on specific parts of the code. As Swift developers, we face many debugging challenges. But with the right tools, like Xcode’s Memory Graph Debugger and LLDB, solving problems becomes easier.
Debugging is key to making sure our Swift apps work well. By using good debugging methods, we fix errors quickly and learn more about our code. Whether it’s quick print statements or detailed memory analysis, each method is important for solving problems efficiently.
Key Takeaways
- Breakpoints allow for specific sections of code to be examined during debugging, aiding in effective error resolution.
- The View Hierarchy Debugger is utilized for visually inspecting the user interface hierarchy of an application in Xcode.
- The Memory Graph Debugger helps in identifying memory leaks and finding retain cycles.
- LLDB, an open-source command-line debugging tool by Apple, is crucial for finding and rectifying errors in Swift applications.
- Log statements are one of the most effective debugging techniques, allowing us to monitor variable values and program flow.
Introduction to Swift Debugging
Learning the basics of *Swift debugging* is key for developers. It’s not just about finding and fixing code errors. It’s about making sure apps work well and perform well. By getting good at *code debugging*, developers can make their software better.
The LLDB debugger, included with Xcode, is a big help for *error diagnosis*. It lets us stop code, check variables, and look at code in detail. This is especially useful when working with third-party frameworks or using continuous integration systems. Also, using LLDB well means knowing how to manage source paths with its commands.
Print statements are also important for *Swift debugging basics*. They let developers see what’s happening in the code quickly. They’re used to print out variable values and more, helping us understand code flow.
Using unit tests with XCTest is another key part of Swift development. Unit tests check if individual parts of code work right. This is crucial for finding exact problems, making debugging easier.
Tools like the *Debug Navigator* in Xcode are also vital. They show us how the program is doing and how it uses memory. Breakpoints let us stop code to examine it closely. These tools help us see how code works and find performance issues.
For those wanting to learn more about Swift debugging, WWDC presentations are great. They teach advanced Xcode and LLDB techniques. Knowing these can help us deal with complex code more efficiently.
Setting Up Your Debugging Environment
Setting up a good debugging environment is key. The first step is to install Xcode, our main tool for Swift development. Knowing how to use the Xcode debugger is also crucial. It helps us check app states, set breakpoints, and step through code.
Installing and Configuring Xcode
Getting Xcode right is the base of a solid Swift debugging setup. Apple’s Xcode gives developers essential debugging tools. After installing, make sure your system meets the requirements and get any extra software needed for debugging.
Configuring Xcode is important for a good debugging experience. Look into Xcode’s Settings and Preferences. Here, you can set up schemes, destinations, and build settings. The Xcode workspace has panels and views for debugging, like the debug navigator and console panel.
Familiarizing with the Debugger
Knowing how to use Xcode’s debugger is vital for Swift developers. The debugger has many features to help with debugging:
- Breakpoints: These pause the program at certain points to check variables and the app’s state.
- Step Over/Into/Out: These tools let you move through code line by line to find problems.
- Variable Inspection: This lets you see and change variable values at runtime, giving insights into the app’s state.
- Logging: The debug console helps monitor runtime logs, helping understand the program’s flow and any unexpected behavior.
Debugging is a big part of software development, often taking more time than writing the code. Mastering these tools is crucial. They help developers work efficiently and solve problems quickly.
By using these features and a systematic debugging approach, we can improve our efficiency. This brings stability and reliability to our Swift apps. For more detailed steps, check out debugging guides and community forums.
Debugging Techniques for Swift
Swift programming has many debugging techniques to find and fix issues quickly. Using good debugging strategies helps us solve problems fast. This makes our apps better for users and more efficient.
Using Print Statements for Quick Debugging
Print statements are a simple way to debug Swift. By adding print statements in our code, we can see how variables change and where the code goes. This helps us find problems easily.
Leveraging Breakpoints
Breakpoints are key for Swift troubleshooting methods. They let us stop the code at certain points to check its state. With conditional breakpoints, we only stop when specific conditions are met. This saves time and boosts efficiency.
Advanced Debugging Tools
For more advanced debugging, tools like Xcode’s Instruments and the Debug Navigator are essential. Xcode has many tools, including:
- LLDB Debugger: A command language for deep debugging.
- View Hierarchy Debugger: Shows views and UI hierarchy for UI debugging.
- Memory Graph Debugger: Finds and fixes memory problems.
- Debug Navigator: Shows memory, network, CPU, disk usage, and call stack.
- Location Simulator: Tests apps in different locations.
Learning about these tools and techniques improves our debugging skills. This makes Swift apps faster and more reliable.
Common Debugging Challenges in Swift
Swift developers often face many challenges. These include common Swift errors, memory leaks, and network debugging. To overcome these, understanding the development environment and using effective strategies is key.
Analyzing Crash Reports
Analyzing crash reports is crucial. They help find the malfunctioning code and identify Swift errors. Crash logs show why apps crash, like nil optionals or out-of-bounds arrays.
By carefully looking at these reports, developers can find and fix the main problem. This makes apps more stable.
Handling Memory Leaks
Memory leaks are a big challenge in Swift. They can make apps use too many resources. To fix this, developers use tools like Instruments.
By using good memory leak solutions, apps run better. Also, using weak and unowned references helps avoid memory leaks.
Troubleshooting Network Requests
Swift network debugging is another challenge. Problems like API issues or server errors can cause network requests to fail. To solve these, developers use tools like Charles Proxy and Postman.
They also use Xcode’s network debugging tools. This helps make sure data is sent correctly, improving user experience.
Debugging Challenge | Tools and Techniques | Impact |
---|---|---|
Analyzing Crash Reports | Crashlytics, Xcode Debugger | Identifies common Swift errors and enhances stability |
Handling Memory Leaks | Instruments, weak/unowned references | Ensures optimal performance and prevents crashes |
Troubleshooting Network Requests | Charles Proxy, Postman, Xcode network debugger | Enhances data transmission efficiency and reliability |
Best Practices for Efficient Swift Debugging
Using Swift debugging best practices can make your software development faster and more reliable. Regular code reviews, unit tests, and clear coding standards are key. Apple’s Unified Logging System helps us manage iOS logs well.
Choosing the right logging tools is important. CocoaLumberjack is great for flexibility and performance. Bugfender helps with real-time bug fixing. NSLogger and SwiftLog also offer useful features like remote logging and a desktop viewer.
Setting up breakpoints well is crucial. The lldb debugger in Xcode lets us pause and check variables. This makes debugging faster by following the run-break-inspect loop.
Knowing how to use logging levels is also important. The Unified Logging System has levels like debug and error. It also protects sensitive info, making debugging stronger.
Debugging Tool | Features | Benefits |
---|---|---|
CocoaLumberjack | Flexibility, performance | Enhanced log management |
Bugfender | Remote logging | Real-time issue diagnosis |
NSLogger | Remote logging, desktop viewer | User-friendly log analysis |
Unified Logging System | Various log levels, privacy controls | Comprehensive logging solution |
Regular unit testing is vital. It makes sure each part works right. This helps fix problems early and makes development smoother.
Conclusion
As we finish our look at efficient debugging in Swift, it’s clear that mastering these skills is key. It helps us make better software. From setting up a good debugging environment in Xcode to using advanced tools, each step is important.
Knowing how to tackle common debugging issues is crucial. This includes using breakpoints, checking for memory leaks, and controlling network speeds. Techniques like working together, switching environments, and managing memory show the value of a varied approach to solving problems.
By using these detailed methods and improving our debugging, we can find and fix bugs better. This makes our apps more stable and efficient. It also helps us stand out as skilled Swift developers. Let’s keep working on these skills and adapt to new challenges in Swift programming.
FAQ
What are some essential debugging techniques for Swift development?
Key debugging techniques in Swift include using print statements for quick insights. You can also set breakpoints for detailed code analysis. Tools like the LLDB debugger and memory graph debugger in Xcode are also helpful.
How do I install and configure Xcode for efficient debugging?
First, download Xcode from the Mac App Store. Then, set up your environment by creating a new project or opening an existing one. It’s important to get familiar with Xcode’s built-in debugger and other tools for efficient debugging.
What is the importance of setting up a debugging environment in Swift?
A good debugging environment helps you find and fix issues quickly. This includes setting up Xcode, understanding the debugger, and using tools like LLDB. These tools are key for inspecting your app’s state and setting breakpoints.
How can print statements be used for quick debugging in Swift?
Print statements let you see the value of variables and the flow of your code. They give you immediate insights into your app, making it easier to find and fix problems.
Why are breakpoints useful in debugging Swift applications?
Breakpoints let you pause your code at specific lines. This way, you can check your app’s state, inspect variables, and control the flow. It gives you deeper insights into bugs.
What are some advanced debugging tools available in Xcode?
Xcode has advanced tools like the LLDB debugger, view hierarchy debugger, and memory graph debugger. These tools help diagnose complex issues and understand memory usage and view layouts.
How can I analyze crash reports effectively in Swift?
To analyze crash reports, look at the data in the crash logs. This helps you find the malfunctioning line of code. It’s key to identifying and fixing the root cause of the crash.
What are some strategies for handling memory leaks in Swift?
To handle memory leaks, use Xcode’s memory graph debugger to find retain cycles. Implement good memory management and ensure objects are deallocated properly.
How can I troubleshoot network requests in a Swift application?
To troubleshoot network requests, check the request and response data and verify network connectivity. Use debugging tools to inspect network traffic. This ensures data transmission is efficient and error-free.
What are some best practices for efficient Swift debugging?
For efficient Swift debugging, do regular code reviews and write unit tests. Maintain a consistent coding standard and use logging strategically. Mastering debugging tools like breakpoints speeds up the process.
Future App Studios is an award-winning software development & outsourcing company. Our team of experts is ready to craft the solution your company needs.