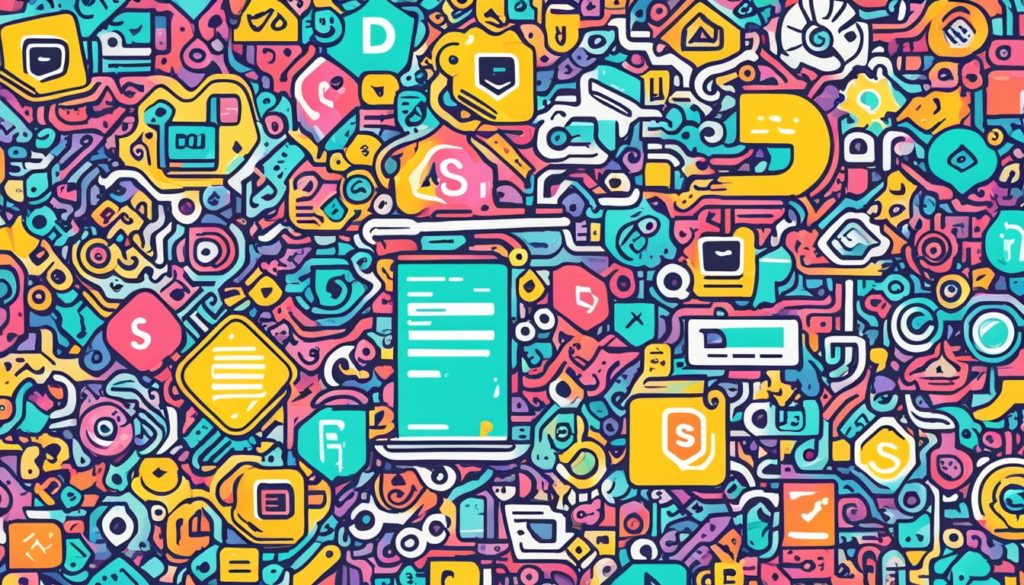
FullCalendar works well with Vue.js to add advanced calendar features in Vue apps. This shows the power of Vue’s component model in making app development easier. We will explore Vue emits, showing how to allow components to talk to each other. This keeps your app easy to manage and work efficiently.
We will dive into the Vue emits method. It’s key for letting components communicate. Imagine making a complex interface where components share data smoothly. Vue.js does this by ensuring data flows one way, keeping things consistent.
Adding FullCalendar to your Vue apps shows Vue.js’s flexibility. FullCalendar, free under the MIT license, gives you strong scheduling features. It fits well with Vue’s system of props and events.
Vue 3’s Composition API has improved how data is emitted in components, making it easier and more structured. This benefits not only experienced developers but also beginners. They learn how to handle component talks and manage Vue.js apps’ state.
Key Takeaways
- The synergy between FullCalendar and Vue.js showcases seamless integration and powerful component interaction.
- Understanding the Vue emits method is crucial for effective parent-child component communication.
- Utilizing the Vue CLI simplifies the setup process for projects involving complex data interactions.
- Vuex state management and Pinia offer robust solutions for handling application-wide state in Vue apps.
- The Composition API in Vue 3 refines event handling, making the logic more explicit and isolated.
Introduction to Vue Emits
Vue.js makes creating web apps easier. It uses emits for component talks. This helps different parts of an app work well together. Getting how emits work is important for good code.
What is Vue Emits?
A child component can tell its parent component something important using Vue emits. Imagine a user clicks a button. The child uses emits to update data the parent controls.
Importance of Component Communication
Apps are made of components that work by themselves. They need to share information clearly. Vue emits helps components work as a team, making apps dynamic and interactive.
When to Use Vue Emits
Knowing when to use Vue emits makes your code clean. It’s best when a child needs to tell a parent component about changes. This makes sure components communicate well in the app’s structure.
Setting Up Vue Emits
To build a great Vue app, getting started right is key. Vue CLI helps with that, making things like auto-compiling easy. It’s great for getting features like hot-module-reloading quickly.
Initial Project Setup with Vue CLI
Let’s start by setting up a new Vue project with Vue CLI. This tool makes installing Vue easy. It sets up our project and handles dependencies for us.
To get Vue CLI, just run:
npm install -g @vue/cli
After installing, create a new project with:
vue create my-project
This command makes a new Vue project in a folder named my-project. It has everything we need to start.
Creating Basic Components
Now that our project is ready, let’s make some basic components. These are essential for any Vue app. We usually keep components in the src/components directory.
To make a new component called MyComponent.vue, just add this code:
<template>
<div>
<h1>Hello from MyComponent!</h1>
</div>
</template>
<script>
export default {
name: 'MyComponent'
}
</script>
This simple component can be added to other parts of our app now.
Overview of Event Handling in Vue
Event handling in Vue is vital for making interactive apps. It helps manage how different parts of the app work together. The $emit
method is crucial for this, especially in Vue emits.
Vue’s event handling lets us make apps that respond to user actions. For instance, a child component can tell a parent component about an action:
<template>
<button @click="notifyParent">Click Me</button>
</template>
<script>
export default {
methods: {
notifyZParent() {
this.$emit('buttonClicked');
}
}
}
</script>
The notifyParent
method lets us send a buttonClicked event. The parent component can respond to this, making our components work well together.
Learning to set up projects with Vue CLI, make components, and handle events gives us a strong start. It helps us build complex features more easily later on.
Using Vue Emits for Parent-Child Communication
Effective Vue.js event communication is crucial for scalable and maintainable apps. In this section, we explore using Vue emits for better parent-child interactions. This approach helps make our Vue.js apps run smoothly.
Emitting Events from Child to Parent
$emit lets child components send signals and data up the hierarchy. This makes Vue apps responsive and interactive. It’s a key pattern in Vue.js.
Developers should use descriptive names for events. This avoids conflicts and makes the code clear.
Handling Emitted Events in Parent Components
Parents must listen and react when children emit an event. Adding event listeners to the child component in the parent’s template makes this easy.
We use v-on or @ to catch emitted events. This maintains a two-way communication channel in our app.
Best Practices and Common Pitfalls in Vue Emits
Following best practices is crucial for effective Vue.js event communication:
- Proper Event Naming: Pick clear, specific names for events to avoid confusion and conflicts.
- One-Way Data Flow: Keep data flow one-way to support Vue’s reactivity. Avoid changing props directly.
- Event Throttling: Throttle events in fast-paced apps to keep performance up.
Avoid mistakes like unscoped emits, which cause confusion in big apps. Handling emitted events well ensures smooth Vue parent-child interactions.
Dynamic Props and Custom Events with Vue Emits
Using dynamic props in Vue helps us pass data in a more lively and efficient way. This makes the connection between components better. We’ll dive into how props change dynamically and the role of custom events in this.
Passing Data with Dynamic Props
Props are the way to send data from a parent component to a child in Vue. Dynamic props offer more than static ones, adding reactivity. This means any updates in the parent’s data are directly shown in the child component.
Creating and Listening to Custom Events
Custom events are a big part of Vue’s flexibility. Through $emit
, a child can tell its parent about important happenings. This is known as Vue emits custom event. It lets developers use the Vue emits listener method for a responsive app.
Examples of Dynamic Data Handling
Vue’s ability to handle dynamic data shows its strength and adaptability. Take form components, for example. They change depending on what a user does and checks for errors. This ensures a user never struggles with sudden changes.
- Binding data dynamically in form inputs
- Adjusting UI components based on state changes
- Utilizing
v-for
for rendering dynamic lists
The combo of dynamic props in Vue and making & listening to custom events lets developers create interactive apps easily.
Advanced Communication Patterns in Vue Emits
Mastering Vue’s advanced communication patterns can make our apps better. They help components work together smoothly. With Vue emits, we can handle difficult situations easily and keep data moving correctly.
Emitting from Nested Child Components
In Vue.js, we often need to chat from deep child components to their parents. Advanced Vue emits let us do this well. They let us send custom events up through the component tree. This keeps our data flow neat and easy to follow.
Using Provide and Inject for Deeply Nested Components
Sometimes, components are deeply tucked away. Provide and inject can make connecting them easier. They let parents give what children need directly. Mixing Vue emits with these makes talking among deep components simple.
State Management with Vuex and Emits
For big apps, Vuex is a lifesaver. It keeps all app data in one place. This makes behavior consistent across components. Vuex works great with Vue emits to manage data changes.
With Vuex, our apps can grow without losing control. It helps handle complex interactions without sweating.
Using Vue’s advanced patterns makes our apps strong and easy to handle. From Vue emits to Vuex, these tools help keep data flowing right. They make sure our apps are scalable and easy to manage.
Conclusion
As we close our discussion on Vue emits, we understand that Vue.js’s way of connecting components is key for creating lively and responsive apps. Vue.js is popular, used in over 2 million websites, due to its simplicity and flexibility. Using Vue emits right lets parent and child components communicate smoothly, keeping code clean and efficient.
Following Vue.js best practices is important. This includes naming custom events in kebab-case and knowing when to use Vuex for state management. With Vue 3’s `defineEmits` and TypeScript, developers can make sure emitted events are correct. This helps create better component documentation.
Understanding the difference between Vue emits and other methods like props is essential. Props are direct and easier to debug, while events are better for sharing functions and logic. This knowledge is vital for anyone wanting to excel at Vue emits, leading to well-organized and scalable apps.
FAQ
What is Vue Emits?
Vue emits allows Vue.js components to send out custom events. This helps parent and child components talk to each other. It makes the code easy to manage.
Why is component communication important in Vue.js?
In Vue.js, it’s key for components to communicate well. This allows the app to work smoothly. Vue emits keep data flowing one way, making it easier to read and debug.
When should I use Vue emits?
Use Vue emits when a child component needs to tell a parent component about something, like when a user clicks a button. It keeps your app’s structure neat and interactive.
How do I set up an initial Vue project using Vue CLI?
Start by installing Vue CLI with npm. Next, create your project with the `vue create` command. This makes setup easy and comes with cool features.
How do I create basic components in Vue?
To make basic components, use the `Vue.component` method or a .vue file. A component has a template, script, and style section.
What is the role of event handling in Vue?
Event handling lets you manage how your app responds to user actions. Vue’s $emit method is important for this, letting child components update parents, making your UI dynamic.
How do I emit events from a child to a parent component?
From a child component, use $emit to send an event. The parent can then catch this event with the v-on directive and react accordingly.
How should I handle emitted events in parent components?
Listen for child events in the parent with v-on or @. Then, respond with methods that update data or trigger actions as needed.
What are some best practices and common pitfalls in using Vue emits?
When using Vue emits, name events clearly and keep data flow one way. Avoid prop mutations and unscoped emits, which can lead to issues in big apps.
How do I pass data with dynamic props in Vue?
Use dynamic props to bind parent data to a child component with v-bind. This keeps the child updated with parent changes, making your app responsive.
How do I create and listen to custom events in Vue?
For custom events, the child component uses $emit. The parent uses v-on to catch these events with a specific handler method.
Can you share examples of dynamic data handling in Vue?
Dynamic data handling in Vue uses props, data binding, and reactivity. For instance, binding a form input to a data property updates the UI with user input instantly.
How do I emit events from nested child components?
To emit events from nested children, chain emits up the component tree. Or, use the provide/inject API to pass data and methods deeper without props.
What is the provide and inject API in Vue?
The provide and inject API lets parents offer data or methods to deep child components. This avoids cluttering the code with props everywhere, making it easier to maintain.
How does Vuex complement Vue emits for state management?
Vuex adds a central store for state management in your app. It works with Vue emits to make sure state changes spread out well, keeping your app scalable and tidy.
Future App Studios is an award-winning software development & outsourcing company. Our team of experts is ready to craft the solution your company needs.