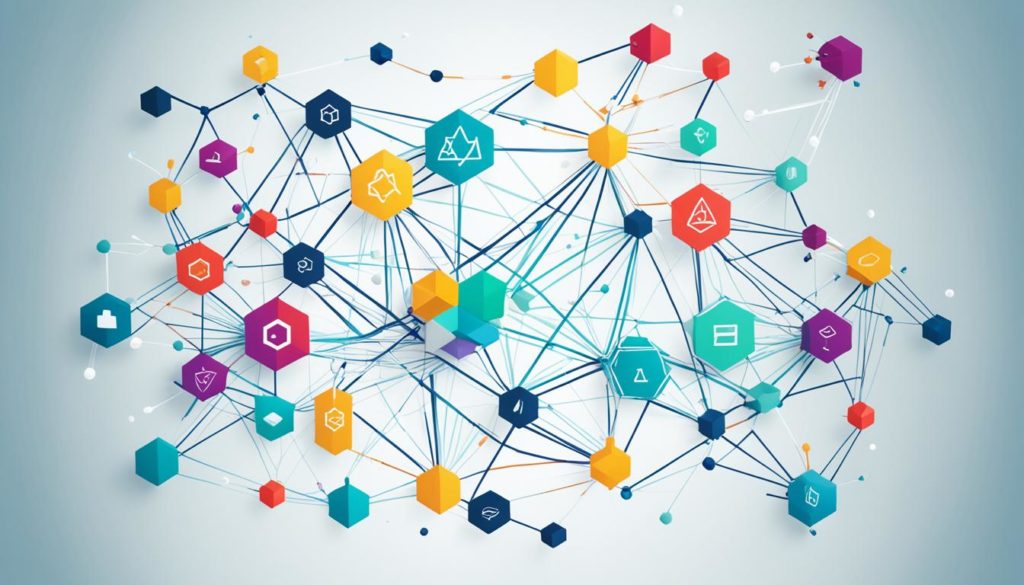
Did you know that using Angular can cut down development time by 40% thanks to its strong Dependency Injection (DI) system? This shows how key it is to understand Angular DI for better software design.
Angular’s Dependency Injection is a key part of its strong framework. It helps us handle our app’s dependencies well, leading to clean code and great type checking. The inject function and constructor-based DI give us many ways to solve different programming problems.
Also, Angular’s updates make DI even more useful. From Angular v14, it works with values, components, directives, and pipes. This makes Angular DI a must-have for developers. Let’s explore how Angular Dependency Injection works and its benefits for our projects.
Key Takeaways
- Angular DI can reduce development time by up to 40%.
- Offers a clean way to manage dependencies within the Angular framework.
- Enhances type inference and code readability with the inject function.
- Angular v14 expands DI functionality to components, directives, and pipes.
- Supports efficient memory management through advanced DI functions.
- Factory providers showcase diverse use cases for dependency creation and injection.
Introduction to Angular Dependency Injection
Dependency injection in Angular is key to making development easier and more efficient. It helps keep our code flexible and easy to test. This method means the framework gives objects what they need instead of the objects making it themselves. This keeps our apps modular and easy to keep up with.
What is Dependency Injection?
Dependency injection (DI) is a way for a class to get its needed dependencies from outside itself. In Angular, DI helps create and manage service instances for us. Using DI, we can add services to components, making our apps more flexible and adaptable.
Importance in Angular Framework
In Angular, DI is crucial for simplifying how we create services and manage their dependencies. It lets developers write clean, organized code where services and components don’t get mixed up. This makes development faster and boosts the reusability and testability of our code.
For more details on how Angular’s dependency injection works, check out this detailed guide on Dependency Injection in Angular.
Feature | Benefit |
---|---|
Loose Coupling | Enhances code maintainability |
Modular Architecture | Improves reusability |
Easy Testing | Simplifies unit testing processes |
Streamlined Dependency Management | Reduces development time |
Understanding the Injector and Injection Tokens
When you work with Angular modules and components, knowing about the injector and injection tokens is key. These concepts are vital for managing dependencies well. Let’s dive into what makes dependency injection so powerful in Angular.
Role of the Injector in Angular
The injector in Angular is a crucial part that makes and keeps services ready. It figures out how to create objects for injection and keeps track of services available. Each injector is like a container that holds service instances. It gives them to Angular components and other classes that need them.
By using Angular modules, the injector manages the lifecycle and scope of dependencies well.
Using Injection Tokens for Dependencies
Injection tokens let you inject custom dependencies without using class types. This is great for non-class data like config objects or simple values. Developers can make their own tokens. These tokens are used as keys to find and register dependencies in the injector.
This method makes Angular components more flexible and helps with managing dependencies better.
Constructor-based DI vs. inject Function
Traditional dependency injection in Angular uses constructors to define dependencies. The injector then fills in these dependencies. This works well but can get tricky with many dependencies or the right order.
The inject function in Angular is a newer way to handle dependencies. It doesn’t need explicit constructors. This makes it easier to manage dependencies, especially in methods and lifecycle hooks.
Dependency Injection Approach | Advantages | Disadvantages |
---|---|---|
Constructor-based DI |
|
|
inject Function |
|
|
Angular Dependency Injection in Practice
Using Dependency Injection (DI) in Angular apps helps us manage services well. It makes sure services are given to Angular components smoothly. These can be built-in, made by us, or from others.
Let’s look at how Angular Dependency Injection works and its benefits:
- Consistency and Modularity: DI makes giving services consistent. This helps our Angular apps be modular and well-organized. We can add services like logging or data getting into any part as needed.
- Improved Testing: With DI, we can easily make fake services for testing. This makes our apps easier to test and keep up with. It’s key for making strong apps.
- Scalability and Maintenance: DI helps our apps grow by making code easier to handle and change. This keeps our apps following good design rules.
For a better grasp, check out guides on injecting services into components. This shows how to use Angular Dependency Injection in real situations.
It’s also key to know the types of DI: constructor, setter, and interface injections. Constructor injection is the most common. But learning about the others gives us more ways to manage dependencies in our Angular apps.
Thinking about these points will show how important DI is. It helps make Angular apps that are easy to keep up with, grow, and are strong.
Optimizing Angular Services with Dependency Injection
Learning about Angular injections is key to making our Angular apps run smoothly. Dependency injection helps us manage services well. It makes sure our code works consistently.
Creating and Injecting Services
In Angular, making and injecting services is easy with Angular providers. The @Injectable decorator, especially with providedIn: ‘root’, lets us create services that are used everywhere. This way, our app runs faster and uses less memory.
For a deeper look at setting up dependency providers in Angular 6.0 and newer, check out this detailed guide on why to set up dependency providers.
Factory Providers and Their Use Cases
Factory providers in Angular are great for making complex services. They’re perfect when we need to use third-party services or adapt services to certain conditions.
Using factory providers lets us add dependencies on the fly. This is super useful when we need different services for different situations. Knowing how to use Angular injections and providers can really improve how we manage services in our apps.
Advanced Techniques with Angular Dependency Injection
We’re going deeper into Angular Dependency Injection. We’ll look at advanced techniques that boost our app’s design and performance. These methods make our design more detailed and strong.
DI Functions: Single Action, Data Provider, Stream Provider
Angular has advanced DI functions like single action, data provider, and stream provider. Each one does a specific job in DI. The single action function does a single task, the data provider gives out data, and the stream provider handles reactive streams well. Using these, developers can make apps that are easier to manage and maintain.
Inheritance and Dependency Injection
Inheritance can make DI harder. But, Angular’s new inject function makes it easier. It lets you inject dependencies straight into subclasses without going through the base classes. This makes the process simpler and makes code easier to read.
Testing and Debugging DI Functions
Testing and debugging Angular DI is key to making sure apps work right. Tools like TestBed and functions like assertInInjectionContext check that DI code works as it should. Knowing how Angular DI works helps developers find and fix problems faster, making sure apps run smoothly.
Conclusion
As we conclude our look at Angular’s Dependency Injection, it’s clear this feature boosts code modularity, reusability, and maintainability. We’ve covered everything from the basics to advanced uses, showing how Angular DI can be used well.
The inject function is a big step forward in Angular development. It offers a flexible and simpler way to use DI compared to older methods. This is especially useful in complex projects and big designs. Using these methods helps developers make their apps better, following Angular DI best practices and keeping up with new trends.
In summary, Angular’s Dependency Injection is more than a framework tool—it makes our code cleaner and easier to maintain. As Angular grows, keeping up with these best practices improves our development work. It makes sure our apps stay strong, efficient, and able to grow. By diving deep into Angular DI, we unlock its full power, making our development work better.
FAQ
What is Dependency Injection in Angular?
Dependency Injection (DI) in Angular means the framework gives classes their needed dependencies. This way, classes don’t make their own dependencies. It makes code easier to test, maintain, and work with.
Why is DI important in the Angular framework?
DI is key in Angular for making app development smoother. It helps manage dependencies well, leads to cleaner code, makes testing easier, and supports code reuse and management.
What is the role of the injector in Angular?
The injector in Angular creates and finds services. It keeps track of how to make services and inject dependencies where needed in the app.
How do Injection Tokens work in Angular?
Injection Tokens in Angular help register and find dependencies. They let developers make custom tokens to inject values or services into different parts of the app.
What is the difference between constructor-based DI and the inject function in Angular?
Constructor-based DI needs to list dependencies in the constructor and can be tricky with many dependencies. The inject function, from Angular v14, makes injecting dependencies easier. It removes the need for constructors and helps with type inference and concise code, especially in class inheritance.
How does one create and inject services in Angular?
To create services in Angular, use the @Injectable decorator. Then, provide them in a module or a component. You can inject them into other components or services using the constructor or the inject function.
What are factory providers, and when should we use them?
Factory providers in Angular create services using a factory function. They’re great for services that need complex setup or when you want different service types under different conditions.
What are DI functions and their usage in Angular?
DI functions in Angular let you do actions, provide data, and manage streams in the injection context. They make code more modular and reusable by offering flexible design patterns.
How does the inject function benefit class inheritance scenarios in Angular?
The inject function makes inheritance easier by avoiding the need to pass all services to base classes. It leads to a cleaner, error-free way to manage dependencies in subclasses.
What are some advanced Angular DI techniques?
Advanced Angular DI includes using DI functions for single actions, data, and stream providers. These methods make designs more flexible and powerful, enhancing app architecture and performance.
How can we test and debug DI in Angular?
Test DI in Angular with TestBed and tools like assertInInjectionContext. These help ensure DI works right and find dependency issues during development.
Future App Studios is an award-winning software development & outsourcing company. Our team of experts is ready to craft the solution your company needs.