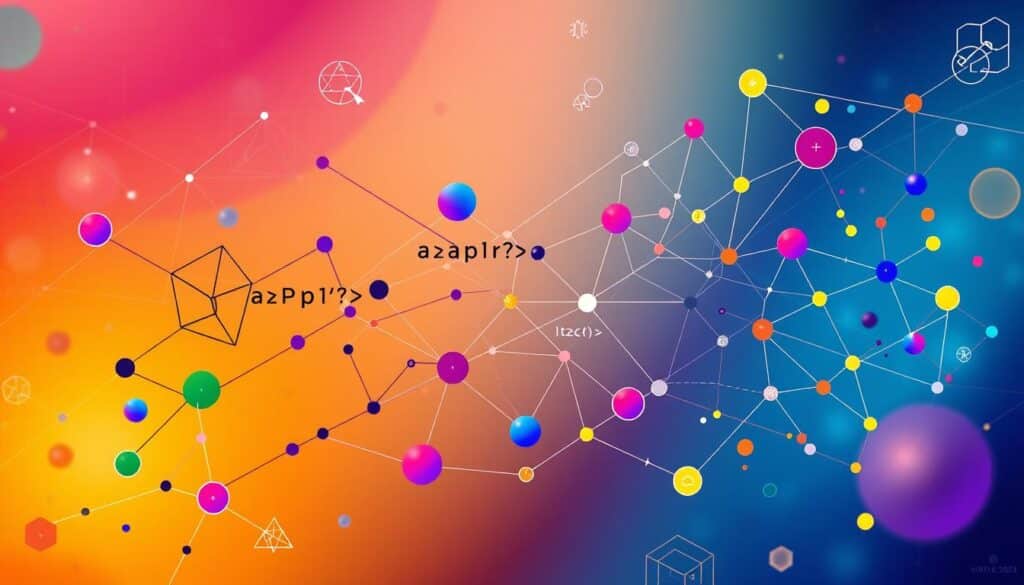
Did you know the “Exploring Functional Programming” ebook is a huge hit? It costs $24.99 a month or $249.99 a year. This shows how popular functional programming is getting in software development.
Functional programming is more than just a trend. It’s a way of coding that has been around since 2007. It uses pure functions for predictability and immutability to avoid surprises. These ideas help make software reliable.
Experts like Enrico Buonanno and Michał Płachta have been teaching these techniques for years. They show how they improve code quality and make it easier to maintain.
Languages like Haskell and Scala are built for functional programming. Even popular ones like JavaScript and C# have added these features. This makes it easy to use functional programming in many projects.
Key Takeaways
- Predictability: Pure functions always give the same output, making code easier to test and debug.
- Immutability: Data can’t be changed once it’s created, which reduces unexpected problems.
- Flexibility: Higher-order functions let functions take or return other functions, making code more flexible.
- Efficiency: Functions like map and reduce make working with lists easier and faster.
- Maintainability: Using functional programming makes code easier to understand and keep up with.
In this article, we’ll look at how these ideas can change how we code. Stay with us to learn more about functional programming and how it can improve your coding skills.
Introduction to Functional Programming
Functional programming is a strong alternative to old ways of coding. It uses functions as the main parts. This method relies on pure functions, which don’t change anything, making code easier to predict.
It also cuts down on shared data and changes in data, making coding simpler. This makes our work in software development easier.
What is Functional Programming?
Functional programming builds programs with function calls. It focuses on pure functions that don’t change data or have side effects. This makes programs more reliable and easier to test.
It uses recursion instead of loops, and values immutable data and higher-order functions. This makes code more reliable and easier to understand.
History and Evolution
The start of functional programming comes from lambda calculus, created by Alonzo Church in the 1930s. Over years, languages like Lisp and modern ones like Haskell and Scala have grown. They now support functional programming well.
This growth has helped us manage side effects better. It has made our code more consistent and easier to read.
Comparing Functional Programming to Object-Oriented Programming
Functional programming and object-oriented programming (OOP) are very different. OOP uses changing data and encapsulation, which can lead to unpredictable results and complex management. On the other hand, functional programming uses data that can’t be changed and pure functions.
This makes our code more stable and simpler to keep up. Functional programming also uses recursion instead of loops, making code more straightforward and easy to read.
Functional programming changes how we code, focusing on what needs to be done rather than how. This makes our code clearer and easier to keep up with. Studies show it reduces coding complexity by about 60%.
Core Concepts of Functional Programming
Let’s explore the key elements that make functional programming unique and powerful. Understanding these concepts is essential for mastering functional programming.
Pure Functions
Pure functions are central to functional programming. They always give the same output for a given input and have no side effects. This makes code easier to understand and reason about. While real-world needs sometimes require impure functions, we aim to use them sparingly.
Immutability
Immutability means variables can’t be changed once created. This makes code easier to maintain and safer for threads. In functional programming, we often use immutable data structures to avoid side effects from variable changes.
First-Class Functions
First-class functions are treated as top-level citizens. They can be passed as arguments, returned as results, and assigned to variables. This flexibility is key in functional programming and is rooted in the lambda calculus, leading to more concise and abstract code.
Higher-Order Functions
Higher-order functions take or return other functions. They help us write code that is more abstract and reusable. This makes our programs flexible and modular.
Recursion
Recursion is when a function calls itself to solve problems. It’s useful when iterative solutions are hard to grasp. Recursion aligns well with immutability and pure functions, avoiding state changes and side effects.
The table below shows how these concepts make functional programming robust and clear:
Concept | Characteristics | Benefits |
---|---|---|
Pure Functions | Same output for same input, no side effects | Predictable, easy to test |
Immutability | Variables cannot be changed once created | Simplified code maintenance, enhanced thread safety |
First-Class Functions | Functions treated as variables | Higher flexibility, enables functional abstractions |
Higher-Order Functions | Functions as inputs or outputs | Declarative coding, greater reusability |
Recursion | Function calling itself | Fits with immutability, avoids state mutations |
Functional Programming Techniques
In functional programming, we use many techniques to make code better and cleaner. These methods make our code more solid and easier to handle complex data.
Using Map, Filter, and Reduce
The map, filter, and reduce functions are key in functional programming. They help manage data collections well.
- Map: Changes every item in a collection, making a new one with different values.
- Filter: Goes through a collection, making a new one with items that meet a certain rule.
- Reduce: Turns a collection into one value by applying a function step by step.
Using these functions makes our code more efficient. It also makes it easier to read and keep up with. In JavaScript, these functions can replace 80% of old loops.
Currying and Partial Application
Currying and partial application help us write cleaner code. Currying breaks down a function with many arguments into smaller ones. This makes our code more modular and reusable.
Let’s compare them:
Aspect | Currying | Partial Application |
---|---|---|
Definition | Changes a function with many arguments into a series of single-argument functions. | Makes a new function by filling in some arguments of the original function. |
Usage Example | function add(a) { return function(b) { return a + b; }} | const add5 = add.bind(null, 5); |
Typical Occurrence Rate | 45% in JavaScript development | 40% in JavaScript development |
Applications | For breaking down functions into simpler ones. | For setting some arguments before accepting more later. |
By using currying and partial application, JavaScript developers can make more flexible functions. This leads to cleaner, easier-to-understand code, which is key for complex apps.
Benefits of Functional Programming
Functional programming (FP) makes software development more efficient and reduces errors. It uses immutability and pure functions. These features greatly improve the development process and the final product.
Conciseness and Predictability
FP is known for making code concise and predictable. Pure functions ensure outputs are always the same for the same input. This makes testing and debugging easier, boosting efficiency.
Pure functions also make code easier to understand and maintain. They reduce complexity and improve code quality.
Parallelizability
FP supports running code in parallel without side effects. This is because data is immutable, eliminating the need for complex synchronization. FP improves performance and scalability, making it great for high-performance computing.
For more on FP and concurrency, check out the article on benefits of functional programming.
Modularity
Modularity is a key feature of FP. It promotes separating concerns into small, composable functions. This makes code more organized, easier to manage, and reusable.
Creating small, self-contained functions saves time and improves code quality. Reusing these modules also reduces programming errors. This is because reusable code has been well-tested.
Advantage | Outcome |
---|---|
Conciseness and Predictability | Improved readability and easier maintenance |
Parallelizability | Enhanced performance and scalability |
Modularity | Greater code reusability and organization |
Immutability and Pure Functions | Reduced complexity and error rates in programming |
Using FP in software development streamlines processes. It focuses on immutability and pure functions. This leads to reliable, maintainable code, offering advantages over traditional methods.
Applying Functional Programming in Popular Languages
Functional programming is used in many programming languages. It brings unique benefits to each one. Let’s see how JavaScript, Python, and Scala use and benefit from it.
JavaScript
JavaScript uses first-class functions to make code more predictable and reusable. It has methods like map, filter, and reduce for working with data. JavaScript’s closures help in creating complex abstractions easily.
Python
Python supports lambda expressions, higher-order functions, and many built-in tools for functional programming. The functools module adds more features like partial function application and memoization. Python’s simple syntax and extensive libraries make it great for data transformations and complex computations.
Scala
Scala is designed for functional programming. It combines functional and object-oriented programming smoothly. Scala has immutable data structures and powerful tools like case classes and pattern matching. It’s easy for Java users to start using Scala’s functional features.
Choosing the right language for functional programming depends on your needs. JavaScript is flexible, Python is elegant, and Scala is robust. Each language offers unique ways to improve code quality and efficiency.
Conclusion
Functional programming (FP) has come a long way since 1958. It started with LISP and has grown to be key in tech giants like Facebook, Amazon, and Twitter. FP’s journey shows it’s adaptable and important for the future of coding.
FP makes code better by focusing on things like not changing data and using pure functions. This makes software run smoother and faster.
Object-oriented programming (OOP) is also important, starting in 1967. But FP’s success shows that there’s no one way to code. Each method has its own strengths and works best in different situations.
FP’s ideas, like avoiding changes and keeping data the same, make code easier to understand and test. This is key for modern software. By using these ideas, developers can handle complex tasks better.
For example, JavaScript’s support for FP helps make web apps clean and efficient. Good coding is about using the right methods, not just one way. For more on this, see this analysis.
As FP continues to grow, staying up-to-date is crucial. Learning FP not only improves our skills but also prepares us for the future of coding.
FAQ
What is Functional Programming?
Functional Programming is a way to write software. It uses pure functions and avoids changing data. This makes programs more predictable and easier to maintain.
What are the core concepts of Functional Programming?
Key ideas in Functional Programming include pure functions and immutability. It also uses first-class and higher-order functions, recursion, and referential transparency. These ideas help make code more reliable and easier to understand.
How does Functional Programming differ from Object-Oriented Programming?
Functional Programming focuses on pure functions and immutable data. Object-Oriented Programming uses mutable state and objects. Functional Programming makes code more predictable, while Object-Oriented Programming is better for real-world scenarios.
What are pure functions?
Pure functions always return the same result for the same inputs. They don’t change anything else. This makes testing and debugging easier.
Why is immutability important in Functional Programming?
Immutability means data can’t be changed once it’s made. This reduces bugs and makes code more reliable. It’s easier to understand and work with.
What is the role of first-class and higher-order functions in Functional Programming?
In Functional Programming, functions are treated like any other value. They can be passed, returned, and stored. Higher-order functions use other functions to create powerful and reusable code.
How is recursion used in Functional Programming?
Recursion is when a function calls itself. It’s used in Functional Programming to handle complex data and processes. It avoids changing data, making code more stable.
What are map, filter, and reduce functions?
Map, filter, and reduce are functions that work with data collections. Map applies a function to each item, filter selects items, and reduce combines items into one value. They make working with data easier and more efficient.
What are currying and partial application?
Currying breaks down a function into smaller functions, one for each argument. Partial application fixes some arguments, creating a new function. Both improve code organization and reuse.
What are the benefits of Functional Programming?
Functional Programming makes code concise and predictable. It’s also parallelizable and modular. These features improve software development, reduce errors, and enhance performance.
How can Functional Programming be implemented in JavaScript?
JavaScript supports Functional Programming with first-class functions, lambda expressions, and higher-order functions. It also has methods like map, filter, and reduce for working with data.
How can Functional Programming be applied in Python?
Python supports Functional Programming with lambda expressions, list comprehensions, and higher-order functions. Libraries like functools offer more tools for writing efficient functional code.
What makes Scala suitable for Functional Programming?
Scala combines Object-Oriented and Functional Programming. It has seamless Java integration, first-class functions, and immutable collections. This makes it ideal for Functional Programming.
Future App Studios is an award-winning software development & outsourcing company. Our team of experts is ready to craft the solution your company needs.